用Vue3-video-play写一个播放组件
时间: 2023-05-30 09:01:13 浏览: 371
Vue3-video-play是一个Vue3的视频播放组件库,可以帮助开发者快速构建视频播放页面。以下是一个使用Vue3-video-play写的视频播放组件:
```vue
<template>
<div class="video-play-wrapper">
<video-player :options="options" ref="player"></video-player>
<div class="video-play-controls">
<div class="video-play-button" @click="togglePlay">
<i v-if="!isPlaying" class="fa fa-play"></i>
<i v-else class="fa fa-pause"></i>
</div>
<div class="video-play-progress-wrapper" @click="updateProgress">
<div class="video-play-progress-bar">
<div class="video-play-progress" :style="{width: progress + '%'}"></div>
</div>
</div>
<div class="video-play-timer">{{ currentTime }} / {{ duration }}</div>
</div>
</div>
</template>
<script>
import { defineComponent, ref, onMounted } from "vue";
import { VideoPlayer } from "vue3-video-play";
export default defineComponent({
components: {
VideoPlayer,
},
props: {
src: {
type: String,
required: true,
},
},
setup(props) {
const player = ref(null);
const options = ref({
sources: [
{
src: props.src,
type: "video/mp4",
},
],
autoplay: false,
controls: false,
});
const isPlaying = ref(false);
const progress = ref(0);
const currentTime = ref("0:00");
const duration = ref("0:00");
const togglePlay = () => {
if (isPlaying.value) {
player.value.pause();
} else {
player.value.play();
}
isPlaying.value = !isPlaying.value;
};
const updateProgress = (event) => {
const progressWrapper = event.target;
const clickX = event.clientX - progressWrapper.getBoundingClientRect().left;
const progressWidth = progressWrapper.offsetWidth;
const percent = (clickX / progressWidth) * 100;
player.value.currentTime = (percent / 100) * player.value.duration;
};
const updateTime = () => {
const currentTimeValue = Math.floor(player.value.currentTime);
const durationValue = Math.floor(player.value.duration);
progress.value = (currentTimeValue / durationValue) * 100;
currentTime.value = formatTime(currentTimeValue);
duration.value = formatTime(durationValue);
};
const formatTime = (time) => {
const minutes = Math.floor(time / 60);
const seconds = time - minutes * 60;
const formattedSeconds = seconds.toString().padStart(2, "0");
return `${minutes}:${formattedSeconds}`;
};
onMounted(() => {
player.value.addEventListener("timeupdate", updateTime);
});
return {
player,
options,
isPlaying,
progress,
currentTime,
duration,
togglePlay,
updateProgress,
};
},
});
</script>
<style>
.video-play-wrapper {
position: relative;
width: 100%;
height: 0;
padding-bottom: 56.25%;
}
.video-play-wrapper video {
position: absolute;
width: 100%;
height: 100%;
}
.video-play-controls {
position: absolute;
bottom: 0;
left: 0;
right: 0;
background-color: rgba(0, 0, 0, 0.5);
display: flex;
align-items: center;
justify-content: space-between;
padding: 8px;
z-index: 1;
}
.video-play-button {
cursor: pointer;
color: white;
font-size: 20px;
}
.video-play-progress-wrapper {
flex: 1;
height: 8px;
margin: 0 8px;
cursor: pointer;
}
.video-play-progress-bar {
height: 100%;
background-color: white;
border-radius: 4px;
overflow: hidden;
}
.video-play-progress {
height: 100%;
background-color: red;
}
.video-play-timer {
color: white;
font-size: 12px;
}
</style>
```
使用方式:
```vue
<template>
<video-play src="https://example.com/video.mp4"></video-play>
</template>
<script>
import VideoPlay from "@/components/VideoPlay.vue";
export default {
components: {
VideoPlay,
},
};
</script>
```
该组件包含了视频播放器和控制条两部分,支持播放、暂停、拖动进度条、显示当前时间和总时间等功能。具体实现细节可见代码注释。
相关推荐
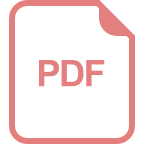
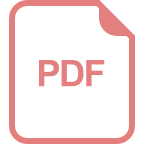














