帮我写一个netty+springboot的tcp的代码
时间: 2023-06-06 17:08:25 浏览: 194
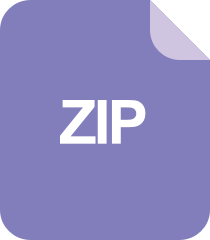
【Java实战】java + netty + springboot 在线聊天室-YadiChat.zip
当然,我很乐意为您提供帮助。以下是一个简单的示例代码,用于创建一个TCP服务器,并通过Netty和Spring Boot框架启动服务器:
```
// 在 pom.xml 文件中添加以下依赖:
// <dependency>
// <groupId>io.netty</groupId>
// <artifactId>netty-all</artifactId>
// <version>4.1.58.Final</version>
// </dependency>
// <dependency>
// <groupId>org.springframework.boot</groupId>
// <artifactId>spring-boot-starter-web</artifactId>
// </dependency>
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import org.springframework.stereotype.Component;
@Component
public class TcpServer {
public TcpServer() {
new Thread(() -> {
NioEventLoopGroup bossGroup = new NioEventLoopGroup();
NioEventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap bootstrap = new ServerBootstrap()
.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new TcpServerHandler());
}
})
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture future = bootstrap.bind(8080).sync();
future.channel().closeFuture().sync();
} catch (Exception e) {
e.printStackTrace();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}).start();
}
}
```
在代码中,我们使用Netty框架创建了一个TCP服务器,并指定服务器要监听的端口(8080)。TcpServerHandler是一个自定义的处理程序,用于处理来自客户端的请求。您可以根据自己的需求修改TcpServerHandler。此外,我们将TcpServer注入Spring容器中,以便在应用程序启动时启动服务器。
阅读全文
相关推荐
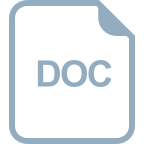
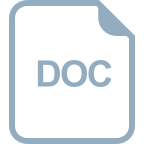
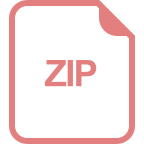
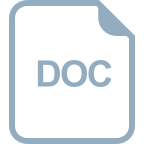
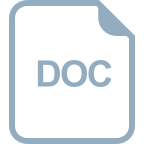
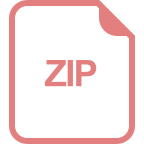
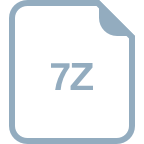
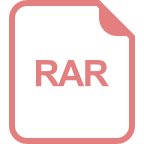
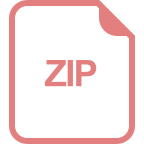
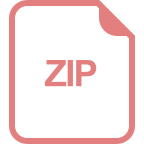
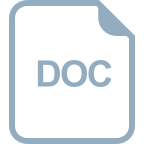
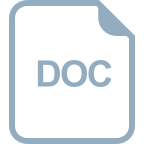
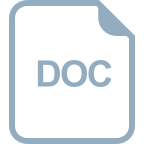
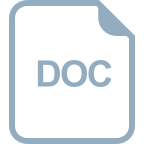
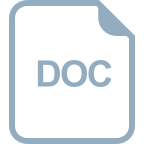



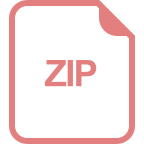