用qt写一段socket代理层的代码实现两个上位机同时连接一个下位机,上位机会向下位机发送命令,两个下位机的数据呈现在上位机上
时间: 2024-03-17 10:42:20 浏览: 121
好的,我会尽力回答你的问题。以下是一个简单的基于Qt的socket代理层代码实现,实现两个上位机同时连接一个下位机,上位机会向下位机发送命令,并将两个下位机的数据呈现在上位机上。
```cpp
#include <QtNetwork>
#include <QTcpServer>
#include <QTcpSocket>
class ProxyServer : public QObject
{
Q_OBJECT
public:
ProxyServer(QObject *parent = 0);
void connectToHost(const QString &hostName, int port);
private slots:
void onClientConnected();
void onClientDisconnected();
void onClientReadyRead();
void onServerConnected();
void onServerDisconnected();
void onServerReadyRead();
private:
QTcpServer *m_proxyServer;
QTcpSocket *m_clientSocket1;
QTcpSocket *m_clientSocket2;
QTcpSocket *m_serverSocket;
};
ProxyServer::ProxyServer(QObject *parent)
: QObject(parent)
{
// Create the proxy server
m_proxyServer = new QTcpServer(this);
connect(m_proxyServer, SIGNAL(newConnection()), this, SLOT(onClientConnected()));
}
void ProxyServer::connectToHost(const QString &hostName, int port)
{
// Create the server socket and connect to the remote host
m_serverSocket = new QTcpSocket(this);
connect(m_serverSocket, SIGNAL(connected()), this, SLOT(onServerConnected()));
connect(m_serverSocket, SIGNAL(disconnected()), this, SLOT(onServerDisconnected()));
connect(m_serverSocket, SIGNAL(readyRead()), this, SLOT(onServerReadyRead()));
m_serverSocket->connectToHost(hostName, port);
}
void ProxyServer::onClientConnected()
{
// Accept the incoming connection from the client
QTcpSocket *clientSocket = m_proxyServer->nextPendingConnection();
// Connect the client socket to the server socket
if (m_clientSocket1 == NULL) {
m_clientSocket1 = clientSocket;
connect(m_clientSocket1, SIGNAL(disconnected()), this, SLOT(onClientDisconnected()));
connect(m_clientSocket1, SIGNAL(readyRead()), this, SLOT(onClientReadyRead()));
} else if (m_clientSocket2 == NULL) {
m_clientSocket2 = clientSocket;
connect(m_clientSocket2, SIGNAL(disconnected()), this, SLOT(onClientDisconnected()));
connect(m_clientSocket2, SIGNAL(readyRead()), this, SLOT(onClientReadyRead()));
}
}
void ProxyServer::onClientDisconnected()
{
// Disconnect the client socket from the server socket
QTcpSocket *clientSocket = qobject_cast<QTcpSocket *>(sender());
if (clientSocket == m_clientSocket1) {
disconnect(m_clientSocket1, SIGNAL(disconnected()), this, SLOT(onClientDisconnected()));
disconnect(m_clientSocket1, SIGNAL(readyRead()), this, SLOT(onClientReadyRead()));
m_clientSocket1 = NULL;
} else if (clientSocket == m_clientSocket2) {
disconnect(m_clientSocket2, SIGNAL(disconnected()), this, SLOT(onClientDisconnected()));
disconnect(m_clientSocket2, SIGNAL(readyRead()), this, SLOT(onClientReadyRead()));
m_clientSocket2 = NULL;
}
}
void ProxyServer::onClientReadyRead()
{
// Forward the client data to the server
QTcpSocket *clientSocket = qobject_cast<QTcpSocket *>(sender());
if (clientSocket == m_clientSocket1) {
m_serverSocket->write(m_clientSocket1->readAll());
} else if (clientSocket == m_clientSocket2) {
m_serverSocket->write(m_clientSocket2->readAll());
}
}
void ProxyServer::onServerConnected()
{
// Server connected
}
void ProxyServer::onServerDisconnected()
{
// Server disconnected
}
void ProxyServer::onServerReadyRead()
{
// Forward the server data to the clients
QByteArray data = m_serverSocket->readAll();
if (m_clientSocket1 != NULL) {
m_clientSocket1->write(data);
}
if (m_clientSocket2 != NULL) {
m_clientSocket2->write(data);
}
}
```
这段代码创建了一个基于Qt的TCP socket代理服务器,它可以同时接受两个上位机的连接,并将它们的数据转发到一个下位机上。使用时,只需创建一个新的ProxyServer对象,然后调用connectToHost()方法连接到下位机的IP地址和端口号。上位机可以通过连接到代理服务器的IP地址和端口号来连接到代理服务器。在代理服务器上,两个上位机的连接将被接受,并将它们的数据转发到下位机上,下位机的数据也将被转发到两个上位机上。
注意:这只是一个简单的示例代码,并没有考虑到错误处理和安全性问题。在实际应用中,请根据实际情况进行修改和完善。
阅读全文
相关推荐
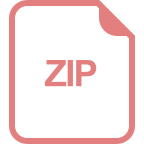
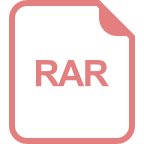




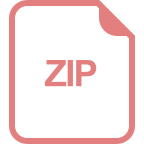
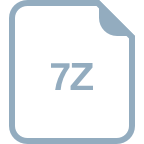
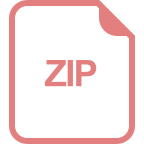
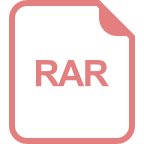