write() argument must be str, not zip
时间: 2024-05-13 14:19:27 浏览: 7
这个错误通常是因为你尝试将一个zip对象传递给了write()函数,而write()函数只接受字符串作为参数。zip()函数是用来压缩多个可迭代对象的函数,它返回一个zip对象,而不是一个字符串。
要解决这个错误,你需要先将zip对象转换成字符串,然后再将其传递给write()函数。你可以使用join()方法将zip对象转换成字符串,例如:
```
my_list = ['a', 'b', 'c']
my_zip = zip(my_list, range(len(my_list)))
my_string = ''.join(str(item) for item in my_zip)
```
这里,我们首先创建了一个列表和一个zip对象。然后,我们使用一个生成器表达式将zip对象转换成字符串,并将其赋值给my_string变量。最后,我们可以将my_string传递给write()函数,而不会出现TypeError异常。
相关问题
write() argument must be str, not generator
This error occurs when you try to pass a generator object as an argument to the built-in `write()` function in Python. The `write()` function is used to write data to a file object or a stream, and it expects a string as its argument.
Here's an example of how this error can occur:
```
# Example 1: Passing a generator to write()
def my_generator():
yield 'Hello'
yield 'world'
with open('output.txt', 'w') as f:
f.write(my_generator()) # ERROR: write() argument must be str, not generator
```
In this example, we define a generator function `my_generator()` that yields two strings: "Hello" and "world". We then try to write the output of this generator to a file using the `write()` function, which results in the error message "write() argument must be str, not generator".
To fix this error, we need to convert the generator output to a string before passing it to `write()`. One way to do this is to use the `join()` method of the string object to concatenate the generator output into a single string:
```
# Example 2: Converting generator output to string before passing to write()
def my_generator():
yield 'Hello'
yield 'world'
with open('output.txt', 'w') as f:
f.write(''.join(my_generator())) # OK: generator output is concatenated into a single string
```
In this example, we use the `join()` method to concatenate the output of the `my_generator()` function into a single string, which we then pass to the `write()` function. This should write the string "Helloworld" to the file "output.txt".
write() argument must be str, not None
This error occurs when you try to pass a None value as an argument to the write() method, which expects a string as input.
To fix this error, make sure that the argument you're passing to the write() method is not None. You can do this by checking the value of the variable or expression you're passing as an argument.
For example:
```
my_string = None
if my_string is not None:
file.write(my_string)
```
In this example, we check if the value of my_string is not None before passing it to the write() method.
Alternatively, you can set a default value for the argument to avoid passing None:
```
def my_function(my_string=None):
if my_string is not None:
file.write(my_string)
```
In this example, we set the default value of my_string to None, but check if it's not None before passing it to the write() method.
相关推荐
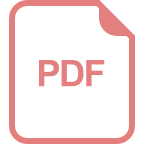
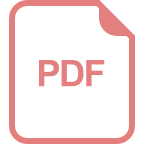
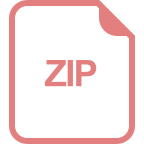












