electron调用python
时间: 2023-07-08 09:47:21 浏览: 91
可以通过两种方式将 Electron 与 Python 集成:
1. 通过 Python Shell 模块:Python Shell 是一个 Node.js 模块,它可以通过子进程执行 Python 脚本。你可以在 Electron 中使用它来调用 Python 脚本。在你的 Electron 项目中安装 Python Shell 模块:
```
npm install python-shell
```
然后在 Electron 中使用它:
```javascript
const {PythonShell} = require('python-shell');
PythonShell.run('my_script.py', null, function (err, results) {
if (err) throw err;
console.log('results: %j', results);
});
```
2. 通过 Python 的网络接口:在 Python 中启动一个 web 服务器,并在 Electron 中使用 Node.js 的 HTTP 模块发送请求。这种方法需要 Python 程序员编写一个 Python web 服务器,以便 Electron 和 Python 之间进行通信。在 Python 中启动 web 服务器:
```python
from http.server import BaseHTTPRequestHandler, HTTPServer
import json
class RequestHandler(BaseHTTPRequestHandler):
def do_POST(self):
content_length = int(self.headers['Content-Length'])
post_data = self.rfile.read(content_length)
json_data = json.loads(post_data.decode('utf-8'))
# 获取传入的参数
arg1 = json_data['arg1']
arg2 = json_data['arg2']
# 调用 Python 函数
result = my_function(arg1, arg2)
# 返回结果
response = {'result': result}
self.send_response(200)
self.send_header('Content-type', 'application/json')
self.end_headers()
self.wfile.write(json.dumps(response).encode('utf-8'))
def run():
httpd = HTTPServer(('localhost', 8080), RequestHandler)
httpd.serve_forever()
if __name__ == '__main__':
run()
```
在 Electron 中调用 Python 函数:
```javascript
const http = require('http');
const postData = JSON.stringify({'arg1': 'hello', 'arg2': 'world'});
const options = {
hostname: 'localhost',
port: 8080,
path: '/',
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Content-Length': Buffer.byteLength(postData)
}
};
const req = http.request(options, (res) => {
res.on('data', (chunk) => {
const result = JSON.parse(chunk)['result'];
console.log(result);
});
});
req.write(postData);
req.end();
```
这两种方法都可以在 Electron 中调用 Python 函数,具体的选择取决于你的需求和技能水平。
相关推荐
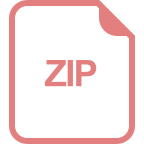
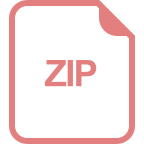
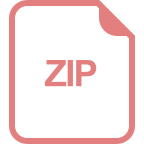














