C 矩阵按键驱动 stm32
时间: 2023-09-02 17:09:05 浏览: 75
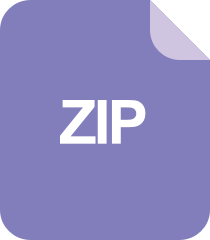
STM32——矩阵按键
以下是一个简单的 C 矩阵按键驱动 stm32 的代码示例:
```c
#include "stm32f10x.h"
#define KEYPAD_PORT GPIOA
#define KEYPAD_ROW1 GPIO_Pin_0
#define KEYPAD_ROW2 GPIO_Pin_1
#define KEYPAD_ROW3 GPIO_Pin_2
#define KEYPAD_ROW4 GPIO_Pin_3
#define KEYPAD_COL1 GPIO_Pin_4
#define KEYPAD_COL2 GPIO_Pin_5
#define KEYPAD_COL3 GPIO_Pin_6
#define KEYPAD_COL4 GPIO_Pin_7
void KEYPAD_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
// 使能IO端口时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
// 配置行为输出模式
GPIO_InitStructure.GPIO_Pin = KEYPAD_ROW1 | KEYPAD_ROW2 | KEYPAD_ROW3 | KEYPAD_ROW4;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_Init(KEYPAD_PORT, &GPIO_InitStructure);
// 配置列为输入模式
GPIO_InitStructure.GPIO_Pin = KEYPAD_COL1 | KEYPAD_COL2 | KEYPAD_COL3 | KEYPAD_COL4;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(KEYPAD_PORT, &GPIO_InitStructure);
}
int KEYPAD_GetKey(void) {
int key = 0;
int i, j;
const int keypad[4][4] = {{1, 2, 3, 10},
{4, 5, 6, 11},
{7, 8, 9, 12},
{14, 0, 15, 13}};
// 扫描按键
for (i = 0; i < 4; i++) {
GPIO_ResetBits(KEYPAD_PORT, KEYPAD_ROW1 << i);
for (j = 0; j < 4; j++) {
if (!GPIO_ReadInputDataBit(KEYPAD_PORT, KEYPAD_COL1 << j)) {
key = keypad[i][j];
while (!GPIO_ReadInputDataBit(KEYPAD_PORT, KEYPAD_COL1 << j))
;
}
}
GPIO_SetBits(KEYPAD_PORT, KEYPAD_ROW1 << i);
}
return key;
}
int main(void) {
int key;
KEYPAD_Init();
while (1) {
key = KEYPAD_GetKey();
if (key) {
// 处理按键事件
}
}
}
```
在这个示例中,我们使用了一个 4x4 的按键矩阵,其中行被配置为输出模式,列被配置为输入模式。在扫描按键时,我们根据行和列的组合来确定按键的编号,并将其返回给主程序进行处理。需要注意的是,由于多个按键可能同时被按下,因此需要进行按键去抖处理。
阅读全文
相关推荐
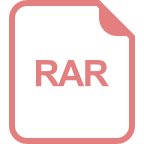
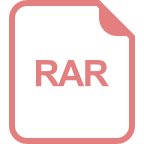
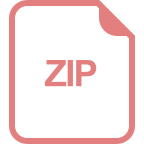
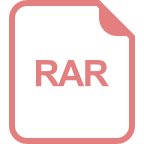
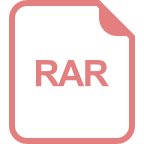
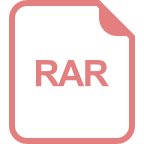
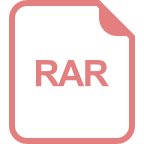
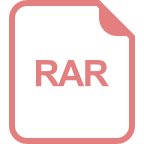

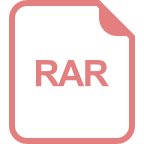
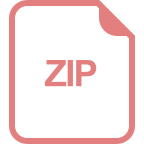
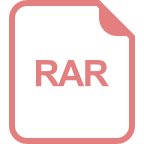
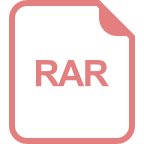
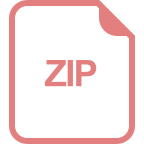
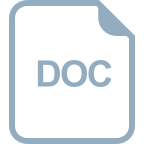
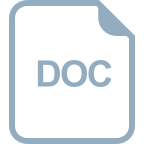