用C语言编写一个程序,将字符数组from中的全部字符拷贝到字符数组to中。要求不使用strcpy函数,拷贝时,'\0'也要拷贝过去,'\0'后面的字符不拷贝。
时间: 2024-03-21 07:40:03 浏览: 32
可以使用循环逐一拷贝字符的方式实现:
```c
#include <stdio.h>
void copy_string(char *to, char *from) {
int i = 0;
while (from[i] != '\0') {
to[i] = from[i];
i++;
}
to[i] = '\0'; // 拷贝 '\0'
}
int main() {
char from[] = "hello, world!";
char to[sizeof(from)];
copy_string(to, from);
printf("from: %s\n", from);
printf("to: %s\n", to);
return 0;
}
```
运行结果:
```
from: hello, world!
to: hello, world!
```
相关问题
c语言编写一个程序将字符数组s2中的全部字符复制到字符数组s1中,不用使用strcpy函数。复制时,'\0'也要拷贝过去。'\0'后面的字符不复制
可以使用循环遍历的方式逐一将字符复制到另一个数组中,直到遇到'\0'为止。以下是示例代码:
```c
#include <stdio.h>
void copyString(char s1[], char s2[]) {
int i = 0;
while (s2[i] != '\0') {
s1[i] = s2[i];
i++;
}
s1[i] = '\0'; // 复制'\0'
}
int main() {
char s1[100], s2[100];
printf("请输入字符串s2:");
scanf("%s", s2);
copyString(s1, s2);
printf("复制后的字符串s1为:%s\n", s1);
return 0;
}
```
在上面的代码中,copyString函数接受两个参数,分别是要复制到的字符串s1和要复制的字符串s2。在函数中,我们使用一个循环来遍历s2中的每个字符,并将其复制到s1中。最后,我们在s1的末尾添加一个'\0',以确保复制的字符串以'\0'结尾。在main函数中,我们首先读入要复制的字符串s2,然后调用copyString函数进行复制,并输出复制后的s1字符串。
编写一个程序,将字符数组s2中的全部字符拷贝到字符数组s1中,不能使用strcpy函数。拷贝时,'\0'也要拷贝过去。C语言
可以使用循环语句逐个复制字符,直到遇到'\0'为止。可以使用以下代码实现:
```c
void copyString(char s1[], char s2[]) {
int i = 0;
while (s2[i] != '\0') { // 逐个复制字符
s1[i] = s2[i];
i++;
}
s1[i] = '\0'; // 复制完后,最后一个字符为'\0'
}
```
使用示例:
```c
#include <stdio.h>
int main() {
char s1[100] = "Hello, World!";
char s2[100] = "This is a test.";
copyString(s1, s2);
printf("s1: %s\n", s1); // 输出:s1: This is a test.
return 0;
}
```
阅读全文
相关推荐
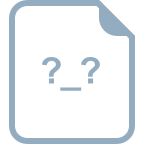
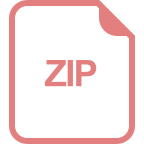
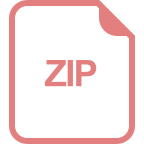










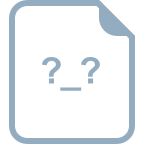
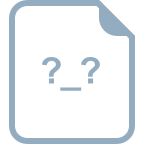