unity控制轮播图的滑动和自动切换图片。
时间: 2024-03-21 09:44:15 浏览: 33
控制轮播图的滑动和自动切换图片可以通过编写脚本来实现。下面是一个简单的示例脚本,可以实现轮播图的自动滑动和手动滑动:
```csharp
using UnityEngine;
using UnityEngine.UI;
public class Carousel : MonoBehaviour
{
public float autoScrollInterval = 3f; // 自动滑动间隔时间
public float scrollSpeed = 100f; // 滑动速度
private ScrollRect scrollRect;
private RectTransform content;
private int currentIndex = 0;
private float timer = 0f;
void Start()
{
scrollRect = GetComponent<ScrollRect>();
content = scrollRect.content;
}
void Update()
{
// 自动滑动
timer += Time.deltaTime;
if (timer >= autoScrollInterval)
{
timer = 0f;
currentIndex = (currentIndex + 1) % content.childCount;
scrollRect.horizontalNormalizedPosition = (float)currentIndex / (content.childCount - 1);
}
// 手动滑动
if (Input.GetMouseButton(0))
{
scrollRect.velocity = new Vector2(Input.GetAxis("Mouse X") * scrollSpeed, 0f);
}
else
{
int targetIndex = Mathf.RoundToInt(scrollRect.horizontalNormalizedPosition * (content.childCount - 1));
float targetPosition = (float)targetIndex / (content.childCount - 1);
scrollRect.horizontalNormalizedPosition = Mathf.Lerp(scrollRect.horizontalNormalizedPosition, targetPosition, Time.deltaTime * 5f);
}
}
}
```
上述脚本实现了轮播图的自动滑动和手动滑动,其中 autoScrollInterval 变量控制自动滑动的间隔时间,scrollSpeed 变量控制手动滑动的速度。在 Update 方法中,首先实现了自动滑动,通过修改 ScrollRect 的 horizontalNormalizedPosition 属性来切换图片。然后实现了手动滑动,通过检测鼠标左键的状态来控制 ScrollRect 的 velocity 属性实现滑动,同时也实现了手指滑动的支持。最后通过 Mathf.Lerp 方法实现了平滑的滑动效果。
相关推荐
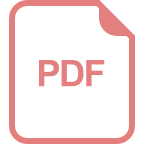
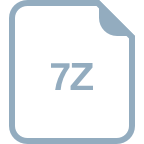
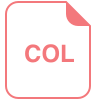













