C语言编写一个简易图书管理系统
时间: 2024-03-20 16:42:02 浏览: 64
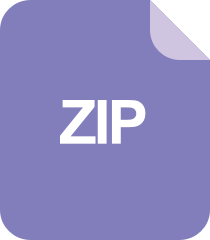
基于C语言的图书管理系统
以下是一个简单的图书管理系统的 C 语言实现,包括添加图书、查找图书、删除图书和显示所有图书的功能。
```c
#include <stdio.h>
#include <string.h>
#define MAX_BOOKS 100
// 图书结构体
struct Book {
char id[10]; // 图书编号
char title[50]; // 图书标题
char author[30]; // 图书作者
int year; // 出版年份
};
// 图书数组
struct Book books[MAX_BOOKS];
int num_books = 0; // 当前图书数量
// 添加图书
void add_book() {
if (num_books >= MAX_BOOKS) {
printf("Sorry, the library is full.\n");
return;
}
struct Book book;
printf("Please enter book ID: ");
scanf("%s", book.id);
printf("Please enter book title: ");
scanf("%s", book.title);
printf("Please enter book author: ");
scanf("%s", book.author);
printf("Please enter publication year: ");
scanf("%d", &book.year);
books[num_books++] = book;
printf("Book added successfully.\n");
}
// 查找图书
void find_book() {
char id[10];
printf("Please enter book ID: ");
scanf("%s", id);
for (int i = 0; i < num_books; i++) {
if (strcmp(id, books[i].id) == 0) {
printf("Book found:\n");
printf("ID: %s\n", books[i].id);
printf("Title: %s\n", books[i].title);
printf("Author: %s\n", books[i].author);
printf("Publication year: %d\n", books[i].year);
return;
}
}
printf("Sorry, book not found.\n");
}
// 删除图书
void delete_book() {
char id[10];
printf("Please enter book ID: ");
scanf("%s", id);
for (int i = 0; i < num_books; i++) {
if (strcmp(id, books[i].id) == 0) {
for (int j = i; j < num_books - 1; j++) {
books[j] = books[j+1];
}
num_books--;
printf("Book deleted successfully.\n");
return;
}
}
printf("Sorry, book not found.\n");
}
// 显示所有图书
void display_books() {
printf("Library contains %d books:\n", num_books);
for (int i = 0; i < num_books; i++) {
printf("ID: %s\n", books[i].id);
printf("Title: %s\n", books[i].title);
printf("Author: %s\n", books[i].author);
printf("Publication year: %d\n", books[i].year);
}
}
int main() {
int choice;
do {
printf("\nLibrary Management System\n");
printf("1. Add book\n");
printf("2. Find book\n");
printf("3. Delete book\n");
printf("4. Display all books\n");
printf("0. Exit\n");
printf("Please enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
add_book();
break;
case 2:
find_book();
break;
case 3:
delete_book();
break;
case 4:
display_books();
break;
case 0:
printf("Goodbye!\n");
break;
default:
printf("Invalid choice.\n");
}
} while (choice != 0);
return 0;
}
```
注意:此为简单的实现,未考虑并发、安全性等问题。在实际开发中需要进行更加严谨的设计和实现。
阅读全文
相关推荐
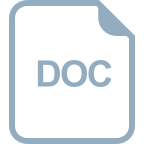
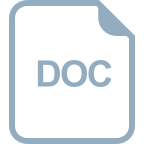
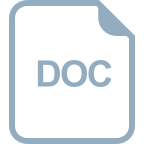
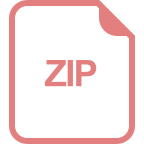
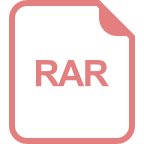
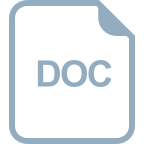


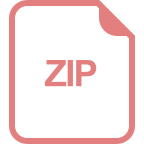
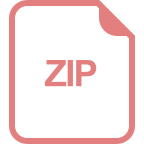
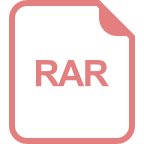
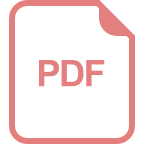
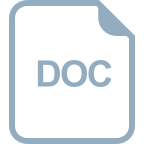
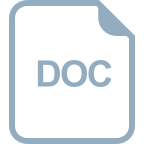
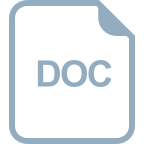
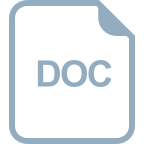
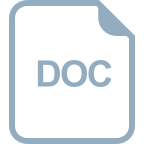
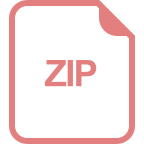