#include <stdio.h> #include <stdlib.h> #include <string.h> int i=0; typedef struct tree{ char data; struct tree *left,*right; }tree; tree* creat(char str[],tree *root){ printf("%c",str[i]); root=malloc(sizeof(tree)); root->data=str[i]; i++; if(root->data=='#'){ root=NULL; } else{ creat(str,root->left); creat(str,root->right); } return root; } int main(void){ char str[80]; while(scanf("%s",&str)!=EOF){ tree *T=NULL; T=creat(str,T); } return 0; }的错误
时间: 2024-03-23 12:39:36 浏览: 20
这段代码的错误在于,在 `creat` 函数内部递归构建树的时候,没有正确处理左右子树的指针。
具体来说,在递归调用 `creat` 函数时,应该将当前节点的左右子树指针分别传递给递归函数,如下所示:
```
creat(str, &(root->left));
creat(str, &(root->right));
```
此外,由于 `scanf` 函数读取字符串时不需要使用 `&`,因此应该将代码中的 `&str` 改为 `str`。
修改后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int i = 0;
typedef struct tree {
char data;
struct tree *left, *right;
} tree;
tree* creat(char str[], tree **root) {
printf("%c", str[i]);
*root = malloc(sizeof(tree));
(*root)->data = str[i];
i++;
if ((*root)->data == '#') {
(*root) = NULL;
} else {
creat(str, &((*root)->left));
creat(str, &((*root)->right));
}
return *root;
}
int main(void) {
char str[80];
while (scanf("%s", str) != EOF) {
tree *T = NULL;
T = creat(str, &T);
}
return 0;
}
```
相关推荐
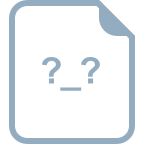
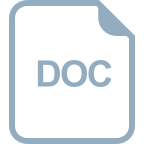
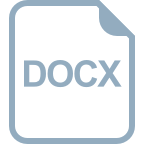















