javahtml转pdf
时间: 2023-10-14 21:06:53 浏览: 29
你可以使用开源的iText库将Java HTML转换为PDF。以下是一个示例代码:
```java
import com.itextpdf.text.Document;
import com.itextpdf.text.DocumentException;
import com.itextpdf.text.PageSize;
import com.itextpdf.text.html.simpleparser.HTMLWorker;
import com.itextpdf.text.pdf.PdfWriter;
import java.io.ByteArrayInputStream;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.nio.charset.StandardCharsets;
import java.util.ArrayList;
import java.util.List;
public class HtmlToPdfConverter {
public static void main(String[] args) throws Exception {
String html = "<html><body><h1>Hello, world!</h1></body></html>";
String pdfPath = "/path/to/pdf/file.pdf";
convertHtmlToPdf(html, pdfPath);
}
public static void convertHtmlToPdf(String html, String pdfPath) throws IOException, DocumentException {
// Create document and writer
Document document = new Document(PageSize.LETTER);
PdfWriter.getInstance(document, new FileOutputStream(new File(pdfPath)));
// Open document
document.open();
// Parse HTML into list of elements
List<com.itextpdf.text.Element> elements = parseHtml(html);
// Add elements to document
for (com.itextpdf.text.Element element : elements) {
document.add(element);
}
// Close document
document.close();
}
private static List<com.itextpdf.text.Element> parseHtml(String html) throws IOException {
// Create input stream from HTML string
InputStream is = new ByteArrayInputStream(html.getBytes(StandardCharsets.UTF_8));
// Parse HTML into list of elements
List<com.itextpdf.text.Element> elements = new ArrayList<>();
HTMLWorker htmlWorker = new HTMLWorker(elements);
htmlWorker.parse(is);
// Return list of elements
return elements;
}
}
```
在上面的示例代码中,我们使用iText库的`Document`和`PdfWriter`类来创建PDF文件。我们还使用了`HTMLWorker`类将HTML字符串解析为iText元素列表。最后,我们将iText元素添加到PDF文档中,并将文档保存到文件系统中。
相关推荐
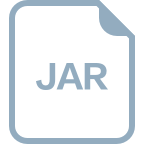

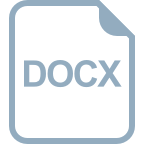
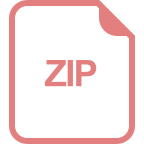
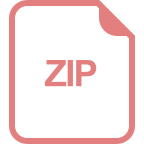
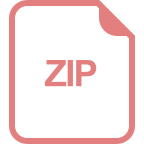
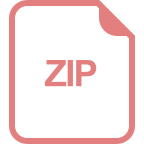
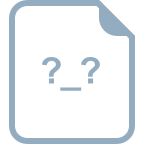
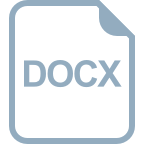
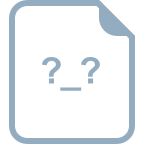