Python画一个可以动爱心
时间: 2023-08-31 19:32:34 浏览: 43
### 回答1:
可以使用 Python 的绘图库来画出一个动态的爱心。
首先,你需要安装一个绘图库,比如说 Matplotlib。你可以使用 `pip` 命令来安装它:
```
pip install matplotlib
```
然后,你可以使用 Matplotlib 的 `animation` 模块来创建动画。首先,你需要导入这个模块:
```python
import matplotlib.pyplot as plt
import matplotlib.animation as animation
```
接下来,你可以使用 Matplotlib 的 `figure` 函数来创建一个新的图形,然后使用 `add_subplot` 函数来添加一个子图,它将作为动画的画布。
```python
fig = plt.figure()
ax = fig.add_subplot(111)
```
然后,你可以定义一个函数来绘制爱心的每一帧。这个函数应该接受一个参数 `i`,表示第 `i` 帧。你可以使用这个参数来控制爱心的形状和位置。
例如,下面的代码使用了 Matplotlib 的 `plot` 函数来绘制一个爱心,并使用 `set_xdata` 和 `set_ydata` 函数来更新爱心的位置:
```python
def draw_heart(i):
x = [0, 0.2, 0.4, 0.6, 0.8, 1, 0.8, 0.6, 0.4, 0.2, 0]
y = [0, 0.2, 0.4, 0.6, 0.8, 1, 0.8, 0.6, 0.4, 0.2, 0]
ax.clear()
ax.plot(x, y)
ax.set_xdata(x)
ax.set_ydata(y)
```
最后,你可以使用 Matplotlib 的 `
### 回答2:
Python是一种功能强大的编程语言,可以用于图形绘制。要画一个可以动的爱心,可以使用Python的turtle模块。下面是一个简单的程序示例:
```python
import turtle
# 设置画笔速度和颜色
turtle.speed(2)
turtle.color('red', 'pink')
# 开始绘制爱心
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
turtle.circle(-90, 200)
turtle.left(120)
turtle.circle(-90, 200)
turtle.forward(224)
turtle.end_fill()
# 窗口保持不关闭
turtle.mainloop()
```
运行以上代码,将会看到一个绘制的爱心图形。如需使爱心动起来,可以使用循环结构来实现。下面是一个实现爱心动画的程序示例:
```python
import turtle
# 设置画笔速度和颜色
turtle.speed(2)
turtle.color('red', 'pink')
# 循环绘制爱心
for i in range(10):
turtle.begin_fill()
turtle.left(140)
turtle.forward(224)
turtle.circle(-90, 200)
turtle.left(120)
turtle.circle(-90, 200)
turtle.forward(224)
turtle.end_fill()
turtle.right(20)
# 窗口保持不关闭
turtle.mainloop()
```
以上程序使用`for`循环结构,绘制了连续的爱心图形,每个爱心稍微旋转一定的角度,从而形成了一个动态的效果。你可以尝试修改上面的代码,调整画笔的速度、颜色和循环次数,以获得不同的效果。希望这个回答对你有所帮助!
### 回答3:
在Python中,我们可以使用turtle模块来绘制一个可以动的爱心。
首先,我们需要导入turtle模块,并创建一个画布和一个画笔对象。
```python
import turtle
canvas = turtle.Screen()
pen = turtle.Turtle()
```
接下来,我们需要定义一个函数来画一个爱心。爱心由两个向外弧线和一个向内弧线组成。我们可以使用turtle模块提供的circle函数来绘制曲线。具体而言,我们可以绘制两段半径不同的圆弧,分别代表爱心的两边,再绘制一个更小的圆弧来连接两边。
```python
def draw_heart():
pen.color("red")
pen.begin_fill()
pen.left(140)
pen.forward(224)
pen.circle(-90, 200)
pen.right(120)
pen.circle(-90, 200)
pen.forward(224)
pen.end_fill()
```
接下来,我们可以调用这个函数来绘制爱心。
```python
draw_heart()
```
最后,我们可以使用一个循环来让爱心动起来。我们可以通过不断改变爱心的位置和角度来实现动画效果。
```python
while True:
pen.penup()
pen.goto(0, 0)
pen.pendown()
pen.right(5)
```
最后,我们只需要在程序结束前调用turtle.done()来保持绘图窗口持续显示。
```python
turtle.done()
```
这样,我们就可以在Python中画一个可以动的爱心了。
完整的代码如下:
```python
import turtle
canvas = turtle.Screen()
pen = turtle.Turtle()
def draw_heart():
pen.color("red")
pen.begin_fill()
pen.left(140)
pen.forward(224)
pen.circle(-90, 200)
pen.right(120)
pen.circle(-90, 200)
pen.forward(224)
pen.end_fill()
draw_heart()
while True:
pen.penup()
pen.goto(0, 0)
pen.pendown()
pen.right(5)
turtle.done()
```
相关推荐
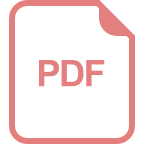
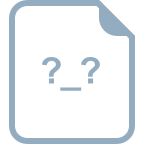
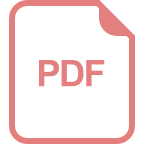
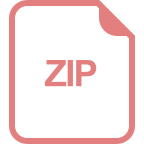
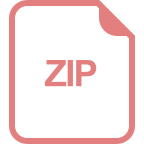
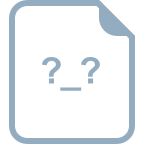
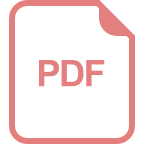
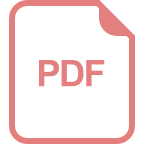
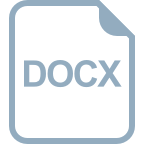
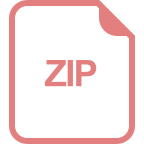