如何实现spark sql 字段血缘分析,请用Java代码实现
时间: 2023-10-17 12:15:01 浏览: 224
要实现 Spark SQL 字段血缘分析,可以通过继承 `org.apache.spark.sql.catalyst.analysis.Analyzer` 类来实现自定义的分析器。下面是一个 Java 版本的实现:
```java
import java.util.HashSet;
import java.util.Set;
import org.apache.spark.sql.catalyst.analysis.Analyzer;
import org.apache.spark.sql.catalyst.expressions.Alias;
import org.apache.spark.sql.catalyst.expressions.Attribute;
import org.apache.spark.sql.catalyst.expressions.Expression;
import org.apache.spark.sql.catalyst.plans.logical.LogicalPlan;
import org.apache.spark.sql.catalyst.plans.logical.Project;
import org.apache.spark.sql.types.DataType;
public class FieldLineageAnalyzer extends Analyzer {
public FieldLineageAnalyzer() {
super();
}
@Override
public LogicalPlan execute(LogicalPlan plan) {
FieldLineageVisitor visitor = new FieldLineageVisitor();
visitor.visit(plan);
return super.execute(plan);
}
private static class FieldLineageVisitor {
private Set<String> currentFields = new HashSet<String>();
public void visit(LogicalPlan plan) {
plan.transformExpressions(expr -> {
if (expr instanceof Attribute) {
Attribute attr = (Attribute) expr;
String fieldName = attr.name();
DataType dataType = attr.dataType();
LineageUtils.addLineage(fieldName, dataType, currentFields);
} else if (expr instanceof Alias) {
Alias alias = (Alias) expr;
Attribute attr = alias.toAttribute();
String fieldName = alias.name();
DataType dataType = attr.dataType();
LineageUtils.addLineage(fieldName, dataType, currentFields);
}
return expr;
});
if (plan instanceof Project) {
Project project = (Project) plan;
currentFields = new HashSet<String>();
for (Expression expr : project.projectList()) {
expr.foreach(attr -> {
if (attr instanceof Attribute) {
String fieldName = ((Attribute) attr).name();
currentFields.add(fieldName);
}
});
}
}
plan.children().forEach(child -> visit(child));
}
}
}
```
这个 `FieldLineageAnalyzer` 类继承了 `Analyzer` 类,并覆盖了 `execute` 方法。在 `execute` 方法中,它首先创建了一个 `FieldLineageVisitor` 实例,并调用它的 `visit` 方法来遍历逻辑计划,执行字段血缘分析。
`FieldLineageVisitor` 类实现了逻辑计划的遍历,并在遍历过程中,使用 `LineageUtils` 类来处理字段血缘关系。在遍历 `Project` 节点时,它会根据 `projectList` 中的表达式来确定当前字段集合。
`LineageUtils` 类用于处理字段血缘关系,它的实现如下:
```java
import java.util.Set;
import java.util.stream.Collectors;
import org.apache.spark.sql.types.DataType;
public class LineageUtils {
private static Set<FieldInfo> fieldInfoSet = new HashSet<FieldInfo>();
public static void addLineage(String fieldName, DataType dataType, Set<String> currentFields) {
FieldInfo fieldInfo = new FieldInfo(fieldName, dataType, currentFields);
fieldInfoSet.add(fieldInfo);
}
public static String getFieldLineage(String fieldName) {
Set<FieldInfo> lineageSet = fieldInfoSet.stream()
.filter(fieldInfo -> fieldInfo.getFieldName().equals(fieldName))
.collect(Collectors.toSet());
Set<String> sourceFields = new HashSet<String>();
for (FieldInfo fieldInfo : lineageSet) {
Set<String> currentFields = fieldInfo.getCurrentFields();
if (currentFields.isEmpty()) {
sourceFields.add(fieldInfo.getFieldName());
} else {
for (String currentField : currentFields) {
String sourceField = getFieldLineage(currentField);
sourceFields.add(sourceField);
}
}
}
return String.join(", ", sourceFields);
}
private static class FieldInfo {
private String fieldName;
private DataType dataType;
private Set<String> currentFields;
public FieldInfo(String fieldName, DataType dataType, Set<String> currentFields) {
this.fieldName = fieldName;
this.dataType = dataType;
this.currentFields = currentFields;
}
public String getFieldName() {
return fieldName;
}
public DataType getDataType() {
return dataType;
}
public Set<String> getCurrentFields() {
return currentFields;
}
}
}
```
在 `LineageUtils` 类中,它定义了一个 `fieldInfoSet` 集合,用于保存字段的详细信息。在 `addLineage` 方法中,它首先构造一个 `FieldInfo` 对象,并将其添加到 `fieldInfoSet` 中。在 `getFieldLineage` 方法中,它根据字段名来查找对应的字段信息,并递归地处理血缘关系,最终返回源字段集合。
这个实现中,字段血缘关系的处理是在遍历逻辑计划的过程中完成的。在遍历每个表达式时,如果它是一个字段或别名,就将其添加到 `LineageUtils` 中。在遍历 `Project` 节点时,它会根据 `projectList` 中的表达式来决定当前字段集合。这样,就可以在执行 Spark SQL 语句时,同时获取到字段血缘关系信息了。
阅读全文
相关推荐
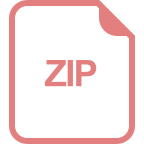

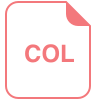
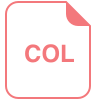
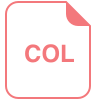
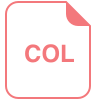

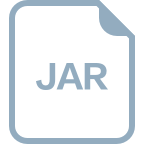
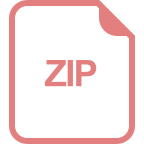
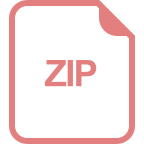
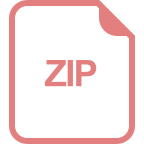
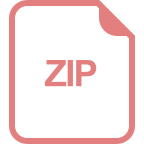