模拟退火算法解决时间窗车辆路径问题
时间: 2023-10-17 14:05:45 浏览: 93
时间窗车辆路径问题是指在一定时间范围内,使得所有客户需求都被满足的情况下,如何合理地安排车辆的路径。模拟退火算法是一种全局优化算法,可以用来解决这种问题。
模拟退火算法的基本思想是模拟物体退火的过程,通过温度不断降低,物体逐渐达到稳定状态。在解决时间窗车辆路径问题中,模拟退火算法可以通过随机交换车辆路径中的节点来寻找更优解。在算法不断迭代的过程中,会以一定的概率接受较差的解,从而避免陷入局部最优解。
具体来说,可以将车辆路径问题转化为一个图论问题,每个节点表示一个客户需求,边表示两个节点之间的距离。然后,随机生成一个初始解,例如每个车辆依次访问所有客户需求。接着,在每一次迭代中,随机交换两个节点,然后计算新解的路径长度,如果新解更优,则接受新解,否则以一定的概率接受较差的解。随着迭代次数的增加,温度逐渐降低,最终得到一个较优的解。
需要注意的是,模拟退火算法的结果并不一定是全局最优解,但是在实际应用中,往往能够得到较好的结果,且算法的时间复杂度相对较低。
相关问题
利用模拟退火算法解决时间窗车辆路径问题的代码
以下是一个简单的利用模拟退火算法求解时间窗车辆路径问题的 Python 代码:
```python
import math
import random
# 车辆路径问题的节点类
class Node:
def __init__(self, x, y, demand, ready_time, due_time, service_time):
self.x = x
self.y = y
self.demand = demand
self.ready_time = ready_time
self.due_time = due_time
self.service_time = service_time
# 计算两个节点之间的距离
def calc_distance(node1, node2):
dx = node1.x - node2.x
dy = node1.y - node2.y
return math.sqrt(dx*dx + dy*dy)
# 计算路径长度
def calc_path_length(path, nodes):
length = 0
cur_time = 0
cur_capacity = 0
for i in range(len(path)):
node = nodes[path[i]]
if cur_capacity + node.demand > capacity:
length += calc_distance(nodes[path[i-1]], nodes[0])
cur_time = max(cur_time + node.service_time, node.ready_time)
cur_capacity = node.demand
else:
cur_time = max(cur_time + calc_distance(nodes[path[i-1]], node) + node.service_time, node.ready_time)
cur_capacity += node.demand
if cur_time > node.due_time:
return float('inf')
if i == len(path) - 1:
length += calc_distance(nodes[path[i]], nodes[0]])
return length
# 模拟退火算法求解路径
def solve(nodes):
# 初始温度、降温系数、迭代次数
T = 100
alpha = 0.99
iterations = 10000
# 初始解
cur_path = list(range(1, len(nodes)))
random.shuffle(cur_path)
# 计算初始解的路径长度
cur_length = calc_path_length(cur_path, nodes)
# 退火过程
while T > 1e-8:
for i in range(iterations):
# 随机交换两个节点
x, y = random.sample(range(len(cur_path)), 2)
new_path = cur_path[:]
new_path[x], new_path[y] = new_path[y], new_path[x]
# 计算新解的路径长度
new_length = calc_path_length(new_path, nodes)
# 根据概率接受新解或者保留当前解
diff = new_length - cur_length
if diff < 0 or random.random() < math.exp(-diff/T):
cur_path = new_path
cur_length = new_length
# 降温
T *= alpha
return cur_path
# 测试代码
capacity = 10
nodes = [Node(0, 0, 0, 0, float('inf'), 0)]
nodes.append(Node(1, 1, 3, 3, 10, 2))
nodes.append(Node(2, 2, 5, 4, 10, 3))
nodes.append(Node(3, 3, 2, 5, 10, 1))
nodes.append(Node(4, 4, 4, 1, 10, 2))
nodes.append(Node(5, 5, 6, 2, 10, 3))
nodes.append(Node(6, 6, 1, 3, 10, 1))
path = solve(nodes)
print(path)
print(calc_path_length(path, nodes))
```
需要注意的是,这只是一个简单的实现,实际应用中需要更加细致地考虑时间窗、容量等限制条件,并进行一些优化。
模拟退火算法求解带时间窗的车辆路径规划问题步骤
模拟退火算法是一种随机优化算法,可以用于求解带时间窗的车辆路径规划问题。其步骤如下:
1. 初始化
随机生成一个初始解,例如随机生成一条路径。
2. 状态转移
对当前解进行随机扰动,例如交换两个节点的位置,得到一个新的解。
3. 接受新解
计算新解的代价函数值,如果新解的代价函数值比当前解的代价函数值更小,则接受新解作为当前解;否则以一定的概率接受新解,以避免陷入局部最优解。
4. 降温
降温是指逐步减小接受新解的概率,以便于算法在搜索到全局最优解时能够收敛。常用的降温策略有线性降温和指数降温。
5. 终止条件
当算法满足终止条件时,停止搜索,并将当前解作为最终解返回。
以上就是模拟退火算法求解带时间窗的车辆路径规划问题的步骤。需要注意的是,模拟退火算法的效果与初始解的质量和降温策略的选择有很大关系,因此需要根据具体问题进行调参。
阅读全文
相关推荐
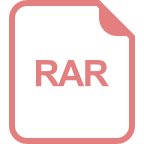
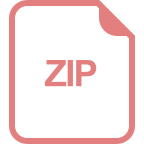
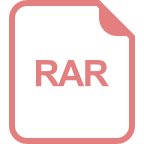













