Scanner scanner1=new Scanner(System.in); String a=scanner1.nextLine(); switch (a) { case "学生": { System.out.println("请输入想要存储的学生人数:"); Scanner scanner = new Scanner(System.in); int number = scanner.nextInt(); List<Student> studentList = new ArrayList<>(); for (int i=0;i<number;i++) { System.out.println("请输入第"+(i+1)+"个人的学号:"); int num = scanner.nextInt(); scanner.nextLine(); System.out.println("请输入第"+(i+1)+"个人的姓名:"); String names = scanner.nextLine(); System.out.println("请输入第"+(i+1)+"个人的所学科目:"); String sub = scanner.nextLine(); Student student = new Student(num, names, sub); studentList.add(student); } for (int i=0;i<number;i++) { System.out.println("第"+(i+1)+"个学生的信息"); System.out.println("学号:"+studentList.get(i).getNumber()); System.out.println("姓名:"+studentList.get(i).getName()); System.out.println("科目:"+studentList.get(i).getSubject()); System.out.println(); } }这串代码如何使用hashmap索引list里面的科目
时间: 2024-02-14 13:04:42 浏览: 29
可以将List<Student>中的每个Student对象的subject作为key,将对应的Student对象作为value存入HashMap<String, Student>中,然后就可以通过key来获取对应的Student对象了。代码如下:
```
Map<String, Student> map = new HashMap<>();
for (Student student : studentList) {
map.put(student.getSubject(), student);
}
```
然后可以通过如下方式来获取对应科目的学生信息:
```
String sub = "数学"; // 假设要获取数学科目的学生信息
Student student = map.get(sub);
if (student != null) {
System.out.println("学号:" + student.getNumber());
System.out.println("姓名:" + student.getName());
System.out.println("科目:" + student.getSubject());
}
```
相关问题
String fileName = new Scanner(System.in).nextLine();
这段代码是用Scanner类从控制台读取一行字符串,并将其存储到fileName变量中。
具体来说,Scanner类是Java中的一个输入类,可以用来读取用户的输入。在这段代码中,通过创建Scanner对象来实现从控制台读取用户输入的功能。nextLine()方法用来读取下一行输入,返回的是一个字符串类型的值,将其存储到fileName变量中。
例如,如果用户在控制台上输入了一个文件名 "test.txt",那么这段代码会将 "test.txt" 字符串存储到 fileName 变量中,以便后续使用。
Scanner sc = new Scanner(System.in);怎么使用?
Scanner类是Java中的一个输入类,用于从控制台或文件中读取输入。使用Scanner类需要先导入java.util包。以下是Scanner类的使用示例:
1. 从控制台读取字符串:
```java
Scanner sc = new Scanner(System.in);
System.out.print("请输入字符串: ");
String str = sc.nextLine();
System.out.println("输入的字符串是: " + str);
```
2. 从控制台读取整数:
```java
Scanner sc = new Scanner(System.in);
System.out.print("请输入整数: ");
int num = sc.nextInt();
System.out.println("输入的整数是: " + num);
```
3. 从控制台读取浮点数:
```java
Scanner sc = new Scanner(System.in);
System.out.print("请输入浮点数: ");
double num = sc.nextDouble();
System.out.println("输入的浮点数是: " + num);
```
4. 从控制台读取布尔值:
```java
Scanner sc = new Scanner(System.in);
System.out.print("请输入布尔值(true/false): ");
boolean b = sc.nextBoolean();
System.out.println("输入的布尔值是: " + b);
```
5. 从控制台读取字符:
```java
Scanner sc = new Scanner(System.in);
System.out.print("请输入字符: ");
char c = sc.next().charAt(0);
System.out.println("输入的字符是: " + c);
```
以上是Scanner类的基本使用方法,可以根据需求进行适当的变化。
相关推荐
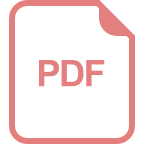
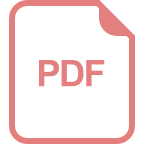
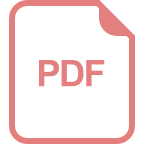










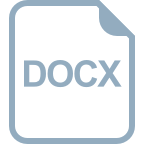
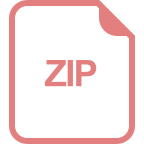
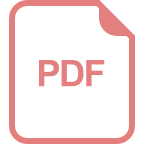
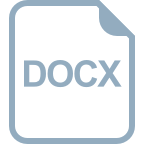