python如何在mysql数据库筛选数据并将结果创建成一个新的mysql数据库
时间: 2024-05-02 18:21:38 浏览: 14
可以使用Python中的pymysql或者MySQLdb模块来连接MySQL数据库,并使用SQL语句来筛选数据和创建新数据库。
以下是一个简单的示例代码:
```python
import pymysql
# 连接到MySQL数据库
conn = pymysql.connect(host='localhost', port=3306, user='root', password='password', db='test')
# 创建游标对象
cursor = conn.cursor()
# 使用SQL语句查询数据
sql = "SELECT * FROM table WHERE column='value'"
cursor.execute(sql)
# 获取查询结果
results = cursor.fetchall()
# 关闭游标和数据库连接
cursor.close()
conn.close()
# 连接到新的MySQL数据库
new_conn = pymysql.connect(host='localhost', port=3306, user='root', password='password', db='new_database')
# 创建游标对象
new_cursor = new_conn.cursor()
# 使用SQL语句创建新数据库表
create_table_sql = "CREATE TABLE new_table (id INT PRIMARY KEY AUTO_INCREMENT, name VARCHAR(255))"
new_cursor.execute(create_table_sql)
# 使用循环将查询结果插入新数据库表中
for result in results:
insert_sql = "INSERT INTO new_table (name) VALUES ('{}')".format(result[1])
new_cursor.execute(insert_sql)
# 提交更改并关闭游标和数据库连接
new_conn.commit()
new_cursor.close()
new_conn.close()
```
这个示例代码连接到MySQL数据库,使用SQL语句查询名为table的表中column为value的行,并将结果存储在变量results中。然后它连接到一个新的MySQL数据库,使用SQL语句创建一个新的表,然后使用循环将查询结果插入新表中。最后,它提交更改并关闭游标和数据库连接。
相关推荐
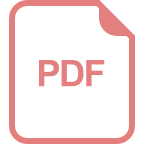
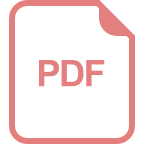
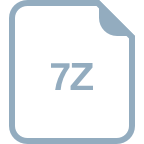














