请使用python实现人脸识别
时间: 2023-12-04 17:57:25 浏览: 100
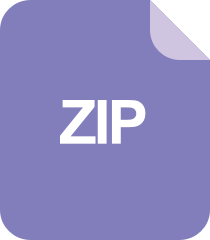
python实现人脸识别
人脸识别需要使用特定的库和算法,以下是一个简单的人脸识别实现代码:
首先,需要安装dlib和OpenCV库:
```python
!pip install dlib
!pip install opencv-python
```
然后,导入所需库:
```python
import cv2
import dlib
import numpy as np
```
接着,加载预训练模型:
```python
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor('shape_predictor_68_face_landmarks.dat')
face_recognition_model = dlib.face_recognition_model_v1('dlib_face_recognition_resnet_model_v1.dat')
```
定义一个函数,用于获取人脸特征向量:
```python
def get_face_encoding(image):
face_locations = detector(image, 1)
shape = predictor(image, face_locations[0])
face_encoding = np.array(face_recognition_model.compute_face_descriptor(image, shape, 1))
return face_encoding
```
现在可以使用上述函数获取两张图片的人脸特征向量,如果两个特征向量很接近,则说明这两张图片中的人是同一个人:
```python
# 加载两张图片
image1 = cv2.imread('image1.jpg')
image2 = cv2.imread('image2.jpg')
# 获取两张图片的人脸特征向量
face_encoding1 = get_face_encoding(image1)
face_encoding2 = get_face_encoding(image2)
# 比较两个特征向量
distance = np.linalg.norm(face_encoding1 - face_encoding2)
if distance < 0.6:
print('这两张图片中的人是同一个人')
else:
print('这两张图片中的人不是同一个人')
```
注意,这只是一个简单的实现,实际应用中还需要考虑人脸姿态、光照等因素的影响,以及更高效、准确的算法。
阅读全文
相关推荐
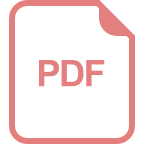
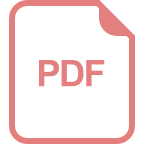







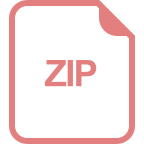
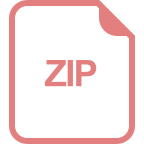
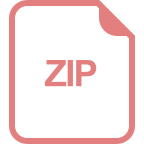
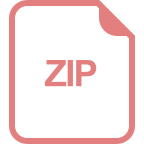
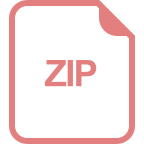
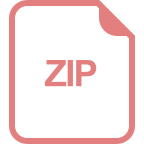