使用Android studio编写读取NT3H2211芯片数据的程序
时间: 2023-05-29 18:06:42 浏览: 182
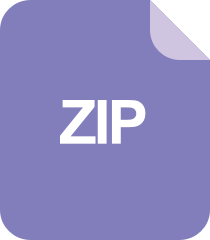
通过Android studio 编写的简易安卓项目

由于NT3H2211芯片是NFC芯片,因此需要使用相关的NFC API来读取芯片数据。以下是一个简单的Android Studio程序,可以读取NT3H2211芯片的NDEF数据:
1. 在build.gradle文件中添加以下依赖项:
```java
dependencies {
...
implementation 'com.android.support:appcompat-v7:28.0.0'
implementation 'com.android.support.constraint:constraint-layout:1.1.3'
implementation 'com.android.support:support-v4:28.0.0'
implementation 'com.android.support:design:28.0.0'
implementation 'com.android.support:cardview-v7:28.0.0'
implementation 'com.android.support:support-core-ui:28.0.0'
implementation 'com.android.support:support-core-utils:28.0.0'
implementation 'com.android.support:support-annotations:28.0.0'
implementation 'com.android.support:support-compat:28.0.0'
implementation 'com.android.support:support-media-compat:28.0.0'
implementation 'com.android.support:support-core-ui:28.0.0'
implementation 'com.android.support:support-core-utils:28.0.0'
implementation 'com.android.support:support-fragment:28.0.0'
implementation 'com.android.support:customtabs:28.0.0'
implementation 'com.android.support:support-vector-drawable:28.0.0'
implementation 'com.google.android.material:material:1.1.0-alpha10'
implementation 'com.android.support:multidex:1.0.3'
implementation 'com.google.android.gms:play-services-nfc:17.0.0'
...
}
```
2. 在AndroidManifest.xml文件中添加以下权限:
```xml
<uses-permission android:name="android.permission.NFC" />
```
3. 在MainActivity.java中添加以下代码:
```java
public class MainActivity extends AppCompatActivity {
private TextView mTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mTextView = findViewById(R.id.text_view);
// 检查设备是否支持NFC
NfcManager nfcManager = (NfcManager) getSystemService(Context.NFC_SERVICE);
NfcAdapter nfcAdapter = nfcManager.getDefaultAdapter();
if (nfcAdapter == null) {
mTextView.setText("该设备不支持NFC!");
return;
}
// 检查NFC是否启用
if (!nfcAdapter.isEnabled()) {
mTextView.setText("请启用NFC功能!");
return;
}
// 设置NFC过滤器
IntentFilter[] intentFilters = new IntentFilter[]{
new IntentFilter(NfcAdapter.ACTION_NDEF_DISCOVERED),
new IntentFilter(NfcAdapter.ACTION_TAG_DISCOVERED),
new IntentFilter(NfcAdapter.ACTION_TECH_DISCOVERED)
};
String[][] techLists = new String[][]{
new String[]{Ndef.class.getName()},
new String[]{MifareClassic.class.getName()},
new String[]{MifareUltralight.class.getName()},
new String[]{NfcA.class.getName()},
new String[]{NfcB.class.getName()},
new String[]{NfcF.class.getName()},
new String[]{NfcV.class.getName()}
};
nfcAdapter.enableForegroundDispatch(this, PendingIntent.getActivity(
getApplicationContext(), 0, new Intent(getApplicationContext(), getClass()), 0),
intentFilters, techLists);
}
@Override
protected void onResume() {
super.onResume();
// 读取NFC标签数据
Intent intent = getIntent();
if (NfcAdapter.ACTION_NDEF_DISCOVERED.equals(intent.getAction())) {
Parcelable[] rawMessages = intent.getParcelableArrayExtra(NfcAdapter.EXTRA_NDEF_MESSAGES);
if (rawMessages != null) {
NdefMessage[] messages = new NdefMessage[rawMessages.length];
for (int i = 0; i < rawMessages.length; i++) {
messages[i] = (NdefMessage) rawMessages[i];
}
byte[] payload = messages[0].getRecords()[0].getPayload();
String text = new String(payload);
mTextView.setText(text);
} else {
mTextView.setText("该NFC标签没有NDEF数据!");
}
} else {
mTextView.setText("请将NFC标签靠近设备!");
}
}
}
```
4. 在activity_main.xml中添加一个TextView:
```xml
<TextView
android:id="@+id/text_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="请将NFC标签靠近设备!"
android:textSize="20sp"
android:textStyle="bold"
android:layout_marginTop="32dp"
android:gravity="center"/>
```
这个程序将在设备检测到NT3H2211芯片时读取芯片中的NDEF数据并在TextView中显示。请注意,您需要将NT3H2211芯片放在设备的NFC天线附近,以确保芯片得到读取。
阅读全文
相关推荐
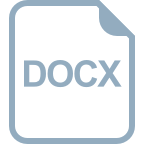






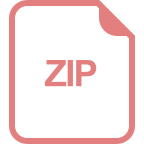
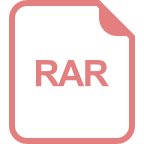
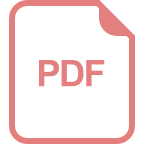
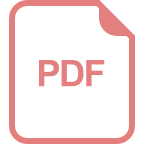
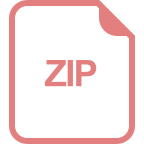
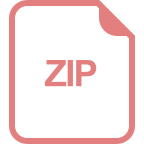



