<script setup> import { computed ,watch } from 'vue'; import { reactive, onMounted } from 'vue'; import { useRouter } from 'vue-router'; import { useStore } from 'vuex'; import { useRoute } from 'vue-router'; // 侧边栏 const items = [ { icon: 'el-icon-ali-home', index: '/dashboard3', title: '系统首页' }, { icon: 'el-icon-ali-cascades', index: '2', title: '储运信息', subs: [ { index: '/logistics', title: '详细信息' } ], }, { icon: 'el-icon-ali-cascades', index: '3', title: '个人管理', subs: [ { index: '/passwordChange3', title: '密码修改' } ], }, ]; const router = useRouter(); watch(router,()=>{ this.reload() }) inject:['reload'] // 路由 const route = useRoute(); watch( () => route.path, (oldValue, newValue) => { // console.log(oldValue, newValue); // removeSearch(); } ); const onRoutes = computed(() => { return route.path; }); const store = useStore(); const collapse = computed(() => store.state.collapse); // 折叠侧边栏 // defineExpose 可以省略 defineExpose({ items, onRoutes, collapse, }); </script> <style scoped> .sidebar { display: block; position: absolute; left: 0; top: 70px; bottom: 0; overflow-y: scroll; } .sidebar::-webkit-scrollbar { width: 0; } .sidebar-el-menu:not(.el-menu--collapse) { width: 250px; } .sidebar > ul { height: 100%; } </style>
时间: 2024-04-27 18:21:54 浏览: 151
这段代码是使用 Vue.js 框架开发的侧边栏组件,其中使用了 Vue.js 提供的 reactive、computed、watch、onMounted 等 API,以及 Vue Router 和 Vuex 等插件。侧边栏的数据由 items 数组提供,每个元素包含图标、索引和标题等属性,如果有子菜单,还包含 subs 属性。另外,该组件还使用了 inject 和 defineExpose API 用于父子组件之间的通信。样式方面,使用了 CSS 控制侧边栏的宽度、位置和滚动等效果。
相关问题
WARN "css.modules" option in vue.config.js is deprecated now, please use "css.requireModuleExtension" instead. INFO Starting development server... 98% after emitting CopyPlugin WARNING Compiled with 17 warnings 09:43:57 warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'computed' was not found in 'vue' warning in ./src/router/index.js "export 'default' (imported as 'VueRouter') was not found in 'vue-router' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'defineComponent' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'getCurrentInstance' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'h' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'inject' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'nextTick' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'onActivated' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'onDeactivated' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'onUnmounted' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'provide' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'reactive' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'ref' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'shallowRef' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'unref' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'watch' was not found in 'vue' warning in ./node_modules/vue-router/dist/vue-router.mjs "export 'watchEffect' was not found in 'vue'这个报错因为什么
这个报错是因为在使用Vue Router时,导入的Vue Router版本与当前项目中的Vue版本不匹配导致的。具体来说,Vue Router中使用了Vue 3的语法而当前项目中使用的是Vue 2,所以会出现一些语法上的错误。解决方法是将Vue Router更新到与当前项目中使用的Vue版本相匹配的版本。
vue3 vue-router 4.1.5 监听route获取不到params
在Vue 3和Vue Router 4.1.5中,你可以通过监听路由来获取参数。但是,在Vue Router 4中,获取参数的方式发生了改变。现在,你需要使用`route`而不是使用`$route`来访问路由参数。
以下是获取路由参数的示例代码:
在Vue组件中,首先需要导入`computed`和`reactive`:
```javascript
import { computed, reactive } from 'vue'
import { useRoute } from 'vue-router'
export default {
setup() {
const route = useRoute()
const params = reactive({
id: computed(() => route.params.id)
})
// 根据需要监听路由的变化,并更新params对象中的值
route.params.id // 通过params对象访问id参数的值
return {
params
}
}
}
```
这样,在Vue 3和Vue Router 4.1.5中,你就可以监听路由并获取到参数的值了。注意,使用`computed`来订阅`route.params`的变化,这样才能实时更新`params`对象中的参数值。
希望这个回答对你有所帮助!
阅读全文
相关推荐
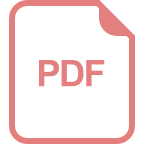
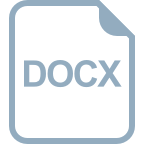
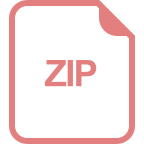
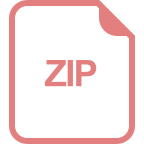
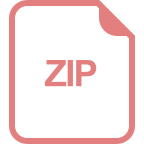
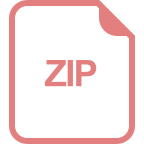
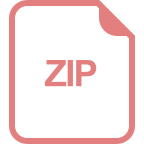
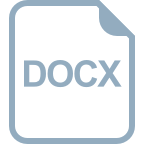
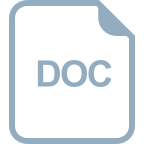
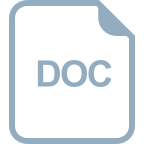
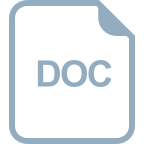
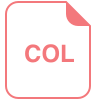



