python 使用随机梯度下降训练鸢尾花代码
时间: 2023-10-24 12:07:56 浏览: 103
下面是使用随机梯度下降(SGD)训练鸢尾花数据集的 Python 代码:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
# 加载数据集
iris = load_iris()
X, y = iris.data, iris.target
# 将类别标签进行 one-hot 编码
y_one_hot = np.zeros((y.size, y.max()+1))
y_one_hot[np.arange(y.size), y] = 1
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y_one_hot, test_size=0.2, random_state=42)
# 定义模型的网络结构
n_input = X_train.shape[1] # 输入层节点数
n_hidden = 5 # 隐藏层节点数
n_output = y_one_hot.shape[1] # 输出层节点数
# 初始化权重和偏置
W1 = np.random.randn(n_input, n_hidden)
b1 = np.zeros(n_hidden)
W2 = np.random.randn(n_hidden, n_output)
b2 = np.zeros(n_output)
# 定义 sigmoid 激活函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义 softmax 激活函数
def softmax(x):
exp_x = np.exp(x)
return exp_x / np.sum(exp_x, axis=1, keepdims=True)
# 定义交叉熵损失函数
def cross_entropy_loss(y_true, y_pred):
return -np.mean(y_true * np.log(y_pred))
# 定义学习率和迭代次数
learning_rate = 0.1
n_iterations = 1000
# 进行模型训练
for i in range(n_iterations):
# 从训练集中随机选择一个样本
idx = np.random.randint(X_train.shape[0])
x = X_train[idx]
y_true = y_train[idx]
# 前向传播
z1 = np.dot(x, W1) + b1
a1 = sigmoid(z1)
z2 = np.dot(a1, W2) + b2
y_pred = softmax(z2)
# 计算损失和梯度
loss = cross_entropy_loss(y_true, y_pred)
delta2 = y_pred - y_true
delta1 = delta2.dot(W2.T) * a1 * (1 - a1)
# 更新权重和偏置
W2 -= learning_rate * a1.reshape(-1, 1).dot(delta2.reshape(1, -1))
b2 -= learning_rate * delta2
W1 -= learning_rate * x.reshape(-1, 1).dot(delta1.reshape(1, -1))
b1 -= learning_rate * delta1
# 在训练集上计算准确率
if i % 100 == 0:
y_pred_train = np.argmax(softmax(X_train.dot(W1).dot(W2)), axis=1)
acc_train = np.mean(y_pred_train == y)
print('Iteration %d, loss=%.4f, train accuracy=%.4f' % (i, loss, acc_train))
```
上面的代码首先加载鸢尾花数据集,将类别标签进行 one-hot 编码,然后将数据集分为训练集和测试集。接着定义模型的网络结构,包括输入层、一个隐藏层和输出层,然后初始化权重和偏置。定义 sigmoid 激活函数、softmax 激活函数和交叉熵损失函数。定义学习率和迭代次数,然后在每次迭代中从训练集中随机选择一个样本进行训练。在每次迭代结束后,在训练集上计算准确率并输出。
阅读全文
相关推荐
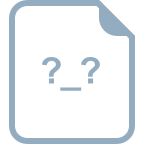
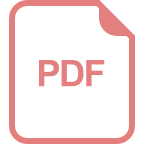
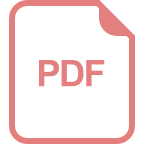
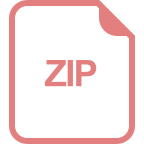
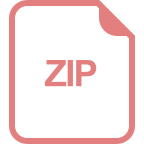