第一行输入若干个关键字,以0作为输入结束
时间: 2024-02-28 22:04:54 浏览: 88
如果你需要在第一行输入若干个关键字,以 0 作为输入结束符,可以使用以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int binary_search(int arr[], int n, int target) {
int left = 0, right = n - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // 没有找到目标元素
}
int main() {
// 输入关键字
int arr[1000], n = 0;
printf("请输入关键字(以 0 结尾):");
while (1) {
int x;
scanf("%d", &x);
if (x == 0) {
break;
}
arr[n++] = x;
}
// 输入目标元素
int target;
printf("请输入目标元素:");
scanf("%d", &target);
// 折半查找
int index = binary_search(arr, n, target);
// 输出结果
if (index == -1) {
printf("关键字中未找到目标元素 %d\n", target);
} else {
printf("目标元素 %d 在关键字中的下标为 %d\n", target, index);
}
return 0;
}
```
在该代码中,首先定义一个长度为 1000 的数组,并使用 `while` 循环输入关键字。当输入数字为 0 时,跳出循环。接下来调用 `binary_search()` 函数查找目标元素,并将结果存储在 `index` 变量中。最后根据 `index` 的值输出结果。
需要注意的是,在这个例子中,我们使用的是 `int` 类型的数组。如果你输入的是字符串等其他类型的数据,需要根据需要进行相应的修改。
阅读全文
相关推荐
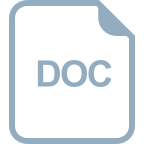
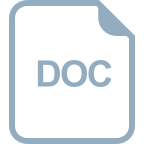
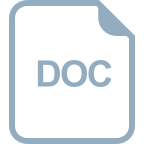








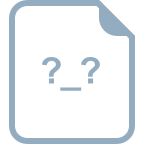
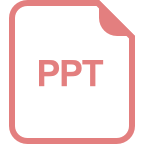
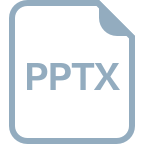
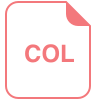
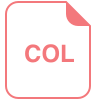
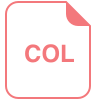
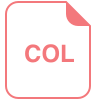
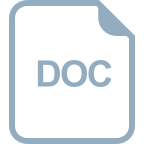