农产品信息管理代码java代码
时间: 2023-07-03 20:24:15 浏览: 33
以下是一个简单的农产品信息管理系统的Java代码示例:
```java
import java.util.Scanner;
class Product {
private String name;
private String type;
private double price;
public Product(String name, String type, double price) {
this.name = name;
this.type = type;
this.price = price;
}
public String getName() {
return name;
}
public String getType() {
return type;
}
public double getPrice() {
return price;
}
public void setName(String name) {
this.name = name;
}
public void setType(String type) {
this.type = type;
}
public void setPrice(double price) {
this.price = price;
}
}
class ProductManager {
private Product[] products;
private int numProducts;
public ProductManager(int numProducts) {
products = new Product[numProducts];
this.numProducts = 0;
}
public void addProduct(Product product) {
if (numProducts < products.length) {
products[numProducts] = product;
numProducts++;
} else {
System.out.println("Product list is full.");
}
}
public void removeProduct(int index) {
if (index >= 0 && index < numProducts) {
for (int i = index; i < numProducts - 1; i++) {
products[i] = products[i + 1];
}
numProducts--;
} else {
System.out.println("Invalid index.");
}
}
public void displayProducts() {
System.out.println("Product List:");
for (int i = 0; i < numProducts; i++) {
System.out.println(i + ". " + products[i].getName() + " (" + products[i].getType() + ") - $" + products[i].getPrice());
}
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
ProductManager productManager = new ProductManager(10);
while (true) {
System.out.println("Select an option:");
System.out.println("1. Add product");
System.out.println("2. Remove product");
System.out.println("3. Display products");
System.out.println("4. Exit");
int option = scanner.nextInt();
if (option == 1) {
System.out.println("Enter product name:");
String name = scanner.next();
System.out.println("Enter product type:");
String type = scanner.next();
System.out.println("Enter product price:");
double price = scanner.nextDouble();
Product product = new Product(name, type, price);
productManager.addProduct(product);
} else if (option == 2) {
System.out.println("Enter index of product to remove:");
int index = scanner.nextInt();
productManager.removeProduct(index);
} else if (option == 3) {
productManager.displayProducts();
} else if (option == 4) {
break;
} else {
System.out.println("Invalid option.");
}
}
}
}
```
这个示例程序使用了一个Product类来表示一个农产品,以及一个ProductManager类来管理所有产品。用户可以选择添加、删除或显示产品,直到选择退出。
相关推荐
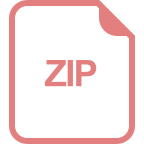
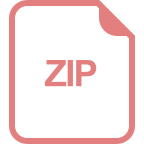
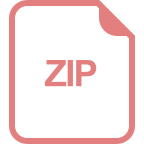












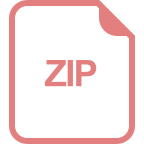
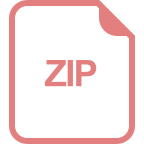
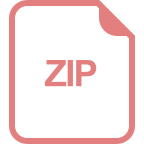