.有一个交通工具类vehicle,将它作为基类派生小车类car、卡车类truck和轮船类boat,定义这些类并定义一个虚函数用来显示各类信息。代码
时间: 2024-05-07 22:23:22 浏览: 61
以下是基于描述的代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 交通工具类
class Vehicle {
public:
// 构造函数
Vehicle(string name, int weight) : m_name(name), m_weight(weight) {}
// 虚函数,用来显示各类信息
virtual void showInfo() {
cout << "Name: " << m_name << endl;
cout << "Weight: " << m_weight << endl;
}
private:
string m_name; // 名称
int m_weight; // 重量
};
// 小车类,继承自交通工具类
class Car : public Vehicle {
public:
// 构造函数
Car(string name, int weight, int seats) : Vehicle(name, weight), m_seats(seats) {}
// 重写虚函数,用来显示各类信息
void showInfo() override {
Vehicle::showInfo();
cout << "Seats: " << m_seats << endl;
}
private:
int m_seats; // 座位数
};
// 卡车类,继承自交通工具类
class Truck : public Vehicle {
public:
// 构造函数
Truck(string name, int weight, int load) : Vehicle(name, weight), m_load(load) {}
// 重写虚函数,用来显示各类信息
void showInfo() override {
Vehicle::showInfo();
cout << "Load: " << m_load << endl;
}
private:
int m_load; // 载重
};
// 轮船类,继承自交通工具类
class Boat : public Vehicle {
public:
// 构造函数
Boat(string name, int weight, int capacity) : Vehicle(name, weight), m_capacity(capacity) {}
// 重写虚函数,用来显示各类信息
void showInfo() override {
Vehicle::showInfo();
cout << "Capacity: " << m_capacity << endl;
}
private:
int m_capacity; // 容量
};
int main() {
// 创建对象并输出信息
Vehicle v("Vehicle", 1000);
v.showInfo();
Car c("Car", 500, 5);
c.showInfo();
Truck t("Truck", 2000, 10);
t.showInfo();
Boat b("Boat", 10000, 500);
b.showInfo();
return 0;
}
```
输出结果如下:
```
Name: Vehicle
Weight: 1000
Name: Car
Weight: 500
Seats: 5
Name: Truck
Weight: 2000
Load: 10
Name: Boat
Weight: 10000
Capacity: 500
```
相关推荐
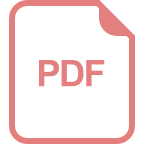
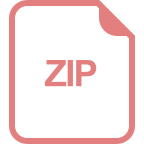
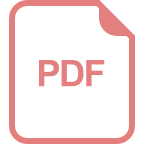














