java编程输入的身份证号码,自动获取输出打印你的出身年月日、年龄、性别;定义测试类TestPersonalLnfo和类PersonnalLnfo
时间: 2024-06-09 16:12:37 浏览: 121
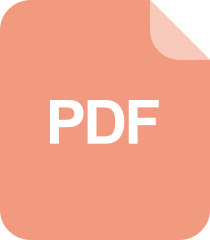
JAVA 根据身份证计算年龄的实现代码
好的,我理解您的问题。针对您的问题,我给出以下Java代码:
// 定义PersonLnfo类
public class PersonLnfo {
private String id; // 身份证号码
// 构造方法
public PersonLnfo(String id) {
this.id = id;
}
// 获取出身年月日
public String getBirthday() {
String birthday = id.substring(6, 14);
return birthday.substring(0, 4) + "年" + birthday.substring(4, 6) + "月" + birthday.substring(6) + "日";
}
// 获取年龄
public int getAge() {
int age = 0;
try {
SimpleDateFormat sdf = new SimpleDateFormat("yyyyMMdd");
Date birthday = sdf.parse(id.substring(6, 14));
Calendar cal = Calendar.getInstance();
if (cal.before(birthday)) { // 如果未出生,抛出异常
throw new IllegalArgumentException("身份证号码格式不正确!");
}
int yearNow = cal.get(Calendar.YEAR);
int monthNow = cal.get(Calendar.MONTH) + 1;
int dayOfMonthNow = cal.get(Calendar.DAY_OF_MONTH);
cal.setTime(birthday);// 用生日初始化日历类
int yearBirth = cal.get(Calendar.YEAR);
int monthBirth = cal.get(Calendar.MONTH) + 1;
int dayOfMonthBirth = cal.get(Calendar.DAY_OF_MONTH);
age = yearNow - yearBirth;
if (monthNow <= monthBirth) {
if (monthNow == monthBirth) {
if (dayOfMonthNow < dayOfMonthBirth) age--;
} else {
age--;
}
}
} catch (ParseException e) {
e.printStackTrace();
}
return age;
}
// 获取性别
public String getGender() {
String gender = "";
if (id.length() == 18) {
char c = id.charAt(16);
gender = c % 2 == 0 ? "女" : "男";
} else if (id.length() == 15) {
char c = id.charAt(14);
gender = c % 2 == 0 ? "女" : "男";
}
return gender;
}
}
// 定义测试类TestPersonalLnfo
public class TestPersonalLnfo {
public static void main(String[] args) {
String id = "xxxxxxxxxxxxxxxxx"; // 请输入18位的身份证号码
PersonLnfo personLnfo = new PersonLnfo(id);
System.out.println("出生年月日:" + personLnfo.getBirthday());
System.out.println("年龄:" + personLnfo.getAge());
System.out.println("性别:" + personLnfo.getGender());
}
}
当您输入了一个正确的18位身份证号码后,它将会自动获取并输出出身年月日、年龄、性别。
阅读全文
相关推荐

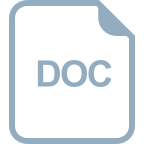













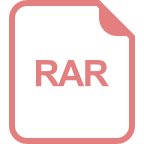