分析封装。 private final String name; private double liquidity; private Set<MarketProperty> portfolio; //constructors //Creating an empty portfolio of assets and zero liquidity. public PropertyManagementCompany(String name, double liquidity) { this.name = checkName(name); this.liquidity = liquidity; this.portfolio = createEmptyPortfolio(); } private Set<MarketProperty> createEmptyPortfolio() { return new TreeSet<>(Comparator.comparingDouble(MarketProperty::getCurrentValuation).reversed()); } //creating a portfolio and liquidity with parameters such as company name, liquidity, and portfolio list public PropertyManagementCompany(String name, double liquidity, List<MarketProperty> portfolio) { this.name = checkName(name); this.liquidity = liquidity; this.portfolio = createEmptyPortfolio(); this.portfolio.addAll(portfolio); } //validators private String checkName(String name) { //The aim of this method is to ensure the type of category. if (name.isEmpty() ) { throw new IllegalArgumentException("The company name can't be empty!"); } else { return name; } } //Purchase a real estate asset with the purchase price. public void buyProperty(MarketProperty property, double price) { if (liquidity >= price) { if (portfolio.contains(property)) { throw new IllegalArgumentException("The property has been held."); } else { //MarketProperty marketProperty = new MarketProperty(property.getID(), property.getCategory(), property.getSize(), property.getInitialPrice()); portfolio.add(property); liquidity -= price; } } else { throw new IllegalArgumentException("Insufficient liquidity to purchase the property. "); } } //Sell a real estate asset with the current valuation. public void sellProperty(MarketProperty property) { if (portfolio.contains(property)) { liquidity += property.getCurrentValuation(); portfolio.remove(property); } else { throw new IllegalArgumentException("Property not found in portfoliio!"); } } //getters public String getName() { return name; } public double getLiquidity() { return liquidity; } public List<MarketProperty> g
时间: 2023-06-18 10:08:23 浏览: 81
This code represents a class named "PropertyManagementCompany" which manages a portfolio of real estate properties. The class has a name, liquidity and a set of properties that it manages. The liquidity represents the amount of money the company has available to purchase new properties or sell existing ones.
The class has two constructors, one that creates an empty portfolio and sets the liquidity to zero, and another that takes a list of properties and adds them to the portfolio. Both constructors also require the company name and liquidity as parameters.
The class has two methods to buy and sell real estate properties. The "buyProperty" method takes a property and a purchase price as parameters and adds the property to the portfolio if the company has enough liquidity to buy it. The "sellProperty" method takes a property and removes it from the portfolio while adding the property's current valuation to the company's liquidity.
The class also has getters for the name, liquidity, and portfolio. The "checkName" method is a private helper method that checks if the company name is empty and throws an exception if it is.
Overall, this code uses encapsulation to hide the implementation details of the PropertyManagementCompany class. The class has private fields that can only be accessed through its public methods. This approach makes the code more modular and easier to maintain.
阅读全文
相关推荐
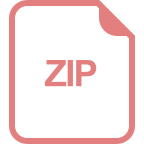
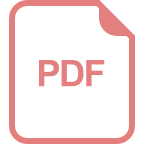
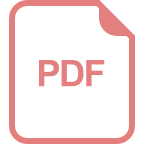
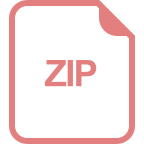
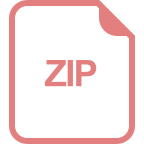
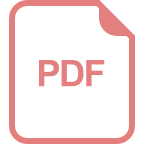
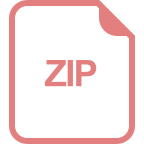
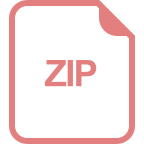
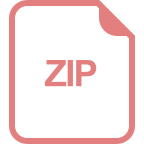
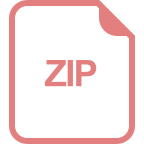
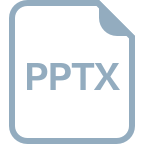
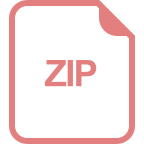
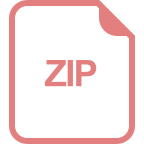
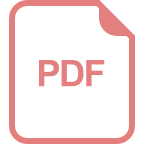
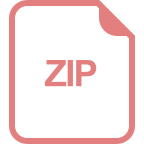
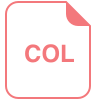


