写一个动态合并单元格前后端代码
时间: 2023-06-14 09:03:49 浏览: 86
前端代码:
```html
<table>
<tbody>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
<tr>
<td rowspan="2">张三</td>
<td>25</td>
<td>男</td>
</tr>
<tr>
<td>30</td>
<td>女</td>
</tr>
<tr>
<td>李四</td>
<td>28</td>
<td>男</td>
</tr>
</tbody>
</table>
```
```javascript
function mergeCells() {
let table = document.getElementsByTagName('table')[0];
let rows = table.rows.length;
let cols = table.rows[0].cells.length;
let markedCells = [];
// 扫描表格,标记需要合并的单元格
for (let i = 0; i < rows; i++) {
for (let j = 0; j < cols; j++) {
let cell = table.rows[i].cells[j];
if (cell.rowSpan > 1 || cell.colSpan > 1) {
markedCells.push({
row: i,
col: j,
rowSpan: cell.rowSpan,
colSpan: cell.colSpan
});
}
}
}
// 合并单元格
for (let i = 0; i < markedCells.length; i++) {
let cell = markedCells[i];
let startRow = cell.row;
let endRow = cell.row + cell.rowSpan - 1;
let startCol = cell.col;
let endCol = cell.col + cell.colSpan - 1;
for (let r = startRow; r <= endRow; r++) {
for (let c = startCol; c <= endCol; c++) {
if (r == startRow && c == startCol) {
continue;
}
table.rows[r].cells[c].style.display = 'none'; // 隐藏单元格
}
}
table.rows[startRow].cells[startCol].rowSpan = cell.rowSpan;
table.rows[startRow].cells[startCol].colSpan = cell.colSpan;
}
}
```
后端代码:
```php
function mergeCells($table) {
$rows = $table->rows->length;
$cols = $table->rows[0]->cells->length;
$markedCells = array();
// 扫描表格,标记需要合并的单元格
for ($i = 0; $i < $rows; $i++) {
for ($j = 0; $j < $cols; $j++) {
$cell = $table->rows[$i]->cells[$j];
if ($cell->rowSpan > 1 || $cell->colSpan > 1) {
$markedCells[] = array(
'row' => $i,
'col' => $j,
'rowSpan' => $cell->rowSpan,
'colSpan' => $cell->colSpan
);
}
}
}
// 合并单元格
foreach ($markedCells as $cell) {
$startRow = $cell['row'];
$endRow = $cell['row'] + $cell['rowSpan'] - 1;
$startCol = $cell['col'];
$endCol = $cell['col'] + $cell['colSpan'] - 1;
for ($r = $startRow; $r <= $endRow; $r++) {
for ($c = $startCol; $c <= $endCol; $c++) {
if ($r == $startRow && $c == $startCol) {
continue;
}
$table->rows[$r]->cells[$c]->style->display = 'none'; // 隐藏单元格
}
}
$table->rows[$startRow]->cells[$startCol]->rowSpan = $cell['rowSpan'];
$table->rows[$startRow]->cells[$startCol]->colSpan = $cell['colSpan'];
}
}
```
阅读全文
相关推荐
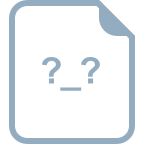


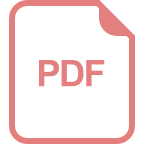
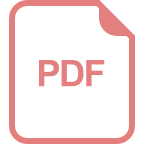
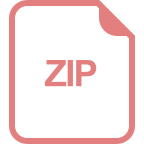
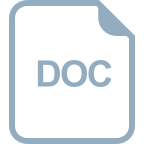
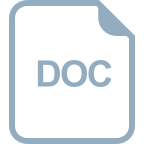
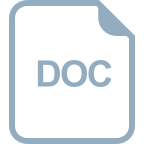
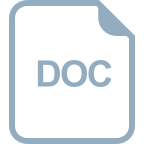
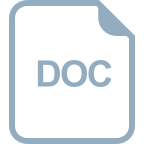
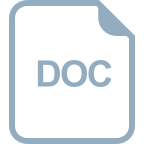
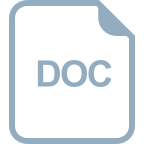
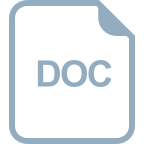
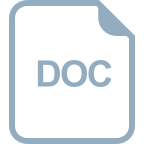

