利用曼哈顿边缘检测,利用c语言,在二值化图像找找矩形,写一份代码
时间: 2023-06-05 19:05:16 浏览: 173
以下是基于曼哈顿边缘检测和c语言的二值化图像矩形查找代码:
```
#include <stdio.h>
#include <stdlib.h>
#define WHITE_PIXEL 255
#define BLACK_PIXEL 0
int is_blank_column(unsigned char *pixels, int height, int width, int column) {
for (int row = 0; row < height; row++) {
if (pixels[row * width + column] == WHITE_PIXEL) {
return 0;
}
}
return 1;
}
int is_blank_row(unsigned char *pixels, int height, int width, int row) {
for (int column = 0; column < width; column++) {
if (pixels[row * width + column] == WHITE_PIXEL) {
return 0;
}
}
return 1;
}
void find_bounding_box(unsigned char *pixels, int height, int width) {
int top, bottom, left, right, count;
top = bottom = left = right = count = 0;
// Find top boundary
for (int row = 0; row < height; row++) {
if (!is_blank_row(pixels, height, width, row)) {
top = row;
break;
}
}
// Find bottom boundary
for (int row = height - 1; row >= 0; row--) {
if (!is_blank_row(pixels, height, width, row)) {
bottom = row;
break;
}
}
// Find left boundary
for (int col = 0; col < width; col++) {
if (!is_blank_column(pixels, height, width, col)) {
left = col;
break;
}
}
// Find right boundary
for (int col = width - 1; col >= 0; col--) {
if (!is_blank_column(pixels, height, width, col)) {
right = col;
break;
}
}
printf("Bounding box: (%d,%d) - (%d,%d)\n", left, top, right, bottom);
}
int main(int argc, char **argv) {
if (argc < 2) {
printf("Usage: %s <image_file>\n", argv[0]);
exit(1);
}
char *image_file = argv[1];
// Load image into memory
FILE *fp = fopen(image_file, "rb");
if (!fp) {
printf("Failed to open %s\n", image_file);
exit(1);
}
int width, height;
unsigned char *pixels;
fscanf(fp, "P5\n%d %d\n255\n", &width, &height);
pixels = (unsigned char *)malloc(width * height * sizeof(unsigned char));
fread(pixels, sizeof(char), width * height, fp);
fclose(fp);
// Threshold image
for (int i = 0; i < width * height; i++) {
pixels[i] = pixels[i] > 127 ? WHITE_PIXEL : BLACK_PIXEL;
}
// Find bounding box
find_bounding_box(pixels, height, width);
free(pixels);
return 0;
}
```
这份代码会读取指定路径的图像文件并把它转换成二值化的像素数据。然后,它会通过曼哈顿边缘检测方法寻找这个矩形的边缘并输出这个矩形的左上角和右下角坐标。
阅读全文
相关推荐
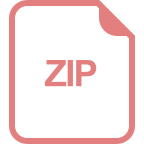
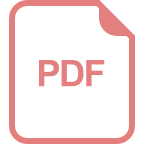
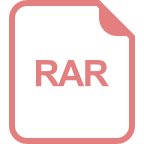
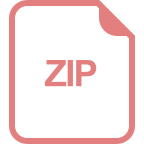
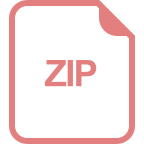
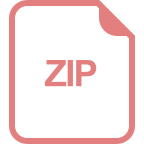
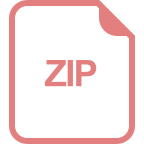
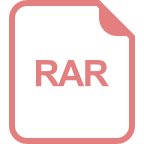
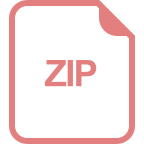
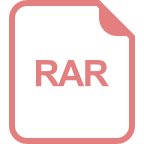
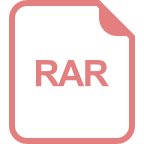
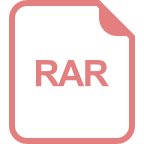
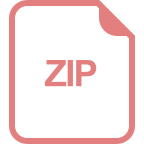
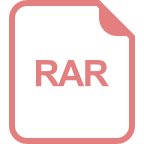
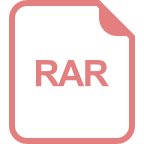
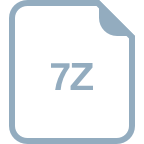