Spring Cloud Alibaba+Spring Boot+Nacos+Dubbo+RocketMQ+Seata+Sentinel
时间: 2023-09-26 22:08:34 浏览: 86
Spring Cloud Alibaba 是阿里巴巴基于 Spring Cloud 推出的一套微服务框架,它提供了一系列的解决方案和工具,帮助开发者快速构建微服务应用。其中,Spring Boot 是基于 Spring 框架的快速开发微服务的工具,Nacos 是一个服务发现和配置中心,Dubbo 是阿里巴巴开源的高性能的 RPC 框架,RocketMQ 是阿里巴巴开源的消息中间件,Seata 是阿里巴巴开源的分布式事务解决方案,Sentinel 是阿里巴巴开源的服务限流和熔断框架。这些技术组合起来,可以帮助开发者快速构建高可用、高性能的微服务应用。
相关问题
使用 Spring Cloud Alibaba+Spring Boot+Nacos+Dubbo+RocketMQ+Seata+Sentinel 搭建项目手脚架
使用 Spring Cloud Alibaba 技术栈搭建项目手脚架可以提高开发效率和项目可维护性,以下是基于 Spring Cloud Alibaba 技术栈的项目手脚架搭建步骤:
1. 环境准备
确保已安装 JDK,Maven,Nacos,RocketMQ,Seata 和 Sentinel 等必需的软件。
2. 创建 Spring Boot 项目
使用 Spring Initializr 创建一个 Spring Boot 项目,添加必需的依赖项:
```xml
<!-- Spring Boot 依赖 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Cloud Alibaba 依赖 -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-config</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-dubbo</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-seata</artifactId>
</dependency>
<!-- RocketMQ 依赖 -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-stream-rocketmq</artifactId>
</dependency>
```
3. 配置 Nacos
在 application.properties 文件中添加 Nacos 相关配置:
```properties
spring.cloud.nacos.discovery.server-addr=127.0.0.1:8848
spring.cloud.nacos.config.server-addr=127.0.0.1:8848
spring.cloud.nacos.config.namespace=dev
```
4. 配置 Dubbo
在 application.properties 文件中添加 Dubbo 相关配置:
```properties
spring.application.name=service-provider
spring.cloud.dubbo.application.name=service-provider
spring.cloud.dubbo.registry.address=nacos://127.0.0.1:8848
spring.cloud.dubbo.protocol.name=dubbo
spring.cloud.dubbo.protocol.port=20880
```
5. 配置 RocketMQ
在 application.properties 文件中添加 RocketMQ 相关配置:
```properties
spring.cloud.stream.rocketmq.binder.name-server=127.0.0.1:9876
spring.cloud.stream.rocketmq.binder.group=group1
```
6. 配置 Seata
在 application.properties 文件中添加 Seata 相关配置:
```properties
spring.cloud.alibaba.seata.tx-service-group=my_test_tx_group
```
7. 配置 Sentinel
在 application.properties 文件中添加 Sentinel 相关配置:
```properties
spring.cloud.sentinel.transport.dashboard=127.0.0.1:8080
```
8. 创建 Dubbo 服务接口
```java
public interface HelloService {
String sayHello(String name);
}
```
9. 实现 Dubbo 服务接口
```java
@Service(version = "1.0.0")
public class HelloServiceImpl implements HelloService {
@Override
public String sayHello(String name) {
return "Hello, " + name;
}
}
```
10. 配置 Dubbo 服务提供者
```java
@Configuration
public class DubboProviderConfig {
@Bean
public ApplicationConfig applicationConfig() {
ApplicationConfig applicationConfig = new ApplicationConfig();
applicationConfig.setName("service-provider");
return applicationConfig;
}
@Bean
public RegistryConfig registryConfig() {
RegistryConfig registryConfig = new RegistryConfig();
registryConfig.setAddress("nacos://127.0.0.1:8848");
return registryConfig;
}
@Bean
public ProtocolConfig protocolConfig() {
ProtocolConfig protocolConfig = new ProtocolConfig();
protocolConfig.setName("dubbo");
protocolConfig.setPort(20880);
return protocolConfig;
}
@Bean
public ProviderConfig providerConfig() {
ProviderConfig providerConfig = new ProviderConfig();
providerConfig.setTimeout(3000);
return providerConfig;
}
@Bean
public ServiceConfig<HelloService> helloServiceServiceConfig() {
ServiceConfig<HelloService> serviceConfig = new ServiceConfig<>();
serviceConfig.setInterface(HelloService.class);
serviceConfig.setRef(new HelloServiceImpl());
serviceConfig.setVersion("1.0.0");
return serviceConfig;
}
}
```
11. 创建测试类
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class HelloServiceTest {
@Reference(version = "1.0.0")
private HelloService helloService;
@Test
public void sayHelloTest() {
String result = helloService.sayHello("World");
Assert.assertEquals("Hello, World", result);
}
}
```
12. 启动项目
正常启动项目,并在浏览器中访问 http://localhost:8848/nacos,可以看到 Nacos 控制台。
在 Dubbo 服务提供者启动后,在 Dubbo 服务消费者中调用 Dubbo 服务,验证服务调用成功。
在 Sentinel 控制台中配置相关规则,验证 Sentinel 报警和限流功能。
在 RocketMQ 控制台中创建一个主题,发送消息,验证消息发送和接收功能。
在 Seata 控制台中创建一个事务分组,验证分布式事务功能。
以上就是使用 Spring Cloud Alibaba 技术栈搭建项目手脚架的步骤,可以根据实际需求进行扩展和修改。
Spring Cloud+Spring Boot+Nacos+Dubbo+mybatis plus+RocketMQ+Seata+Sentinel
Spring Cloud是一个基于Spring Boot的微服务框架,它提供了一整套服务治理的解决方案,包括服务注册与发现、服务调用、负载均衡、断路器、配置管理等功能。
Nacos是阿里巴巴开源的一款服务注册和配置中心,它提供了服务发现、服务健康检查和动态配置等功能,可以与Spring Cloud集成,为微服务架构提供服务注册与发现的能力。
Dubbo是阿里巴巴开源的一款高性能、轻量级的RPC框架,它提供了服务治理的完整解决方案,包括服务注册与发现、负载均衡、容错、服务降级等功能。
Mybatis Plus是Mybatis的增强工具,它提供了许多便捷的查询方式和自动生成代码的功能,可以大大提高开发效率。
RocketMQ是阿里巴巴开源的一个分布式消息队列系统,它提供了可靠的消息传输、高可用性、高吞吐量等特性,可以用于实现异步消息通信和解耦系统之间的依赖关系。
Seata是阿里巴巴开源的一个分布式事务解决方案,它提供了全局事务管理、分布式锁、数据源代理等功能,可以保证分布式系统的数据一致性和事务的可靠性。
Sentinel是阿里巴巴开源的一个流量控制和服务降级框架,它提供了实时监控、流量控制、熔断降级等功能,可以保证系统的稳定性和可靠性。
相关推荐
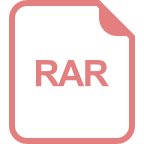
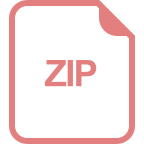
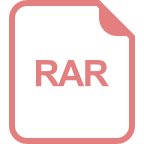












