基于STM32F103ZET6的温湿度串口程序
时间: 2024-05-11 20:18:31 浏览: 111
以下是基于STM32F103ZET6的温湿度串口程序的示例代码:
```
#include "stm32f10x.h"
#include "stdio.h"
#define USART1_DR_Base 0x40013804
void USART1_Init(void);
void USART1_SendChar(unsigned char ch);
void delay_ms(u16 nms);
void delay_us(u32 nus);
int main(void)
{
USART1_Init();
while(1)
{
USART1_SendChar(0x55); //发送读取温湿度的命令
delay_ms(1000); //延时等待传感器响应
u8 buf[6];
u8 i;
for(i=0; i<6; i++)
{
while(!(USART1->SR & USART_SR_RXNE)); //等待接收数据
buf[i] = USART1->DR;
}
float temp = (float)((buf[0]<<8)|buf[1])/10.0; //计算温度值
float humi = (float)((buf[3]<<8)|buf[4])/10.0; //计算湿度值
printf("Temp = %.1fC, Humi = %.1f%%\r\n", temp, humi);
delay_ms(5000); //延时5秒再次读取温湿度
}
}
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
//USART1_TX PA.9
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//USART1_RX PA.10
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(unsigned char ch)
{
while(!(USART1->SR & USART_SR_TXE)); //等待发送缓冲区为空
USART1->DR = (ch & 0xFF);
}
void delay_ms(u16 nms)
{
u16 i, j;
for(i=0; i<nms; i++)
for(j=0; j<1000; j++);
}
void delay_us(u32 nus)
{
u32 i, j;
for(i=0; i<nus; i++)
for(j=0; j<10; j++);
}
```
该程序的主要功能是读取连接在USART1串口上的温湿度传感器的数据,并将温度和湿度值通过串口发送到电脑上显示出来。
该程序使用了STM32F10x标准库,其中USART1被配置为9600波特率、8位数据位、1位停止位、无校验位、无硬件流控制,同时PA.9和PA.10被配置为USART1的TX和RX引脚。
在主函数中,程序首先发送读取温湿度的命令0x55,然后等待传感器响应,接着读取传感器返回的6个字节的数据,并计算出温度和湿度值。最后通过printf函数将温度和湿度值输出到串口上,并延时5秒再次读取温湿度。
阅读全文
相关推荐
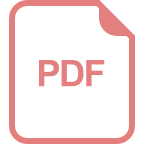
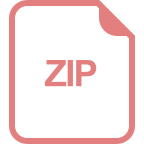
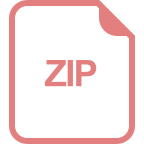
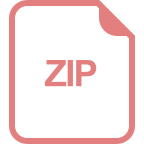

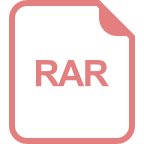
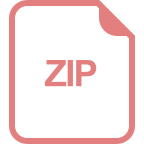
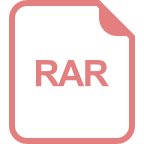
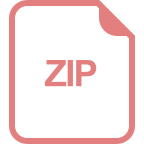
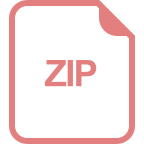
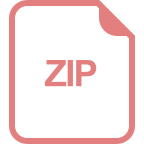
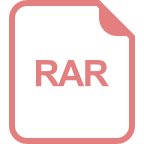
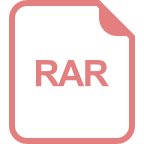
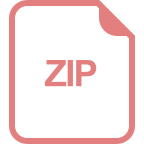
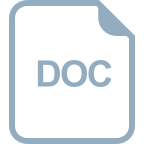
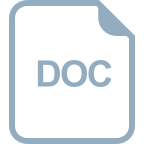
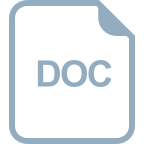
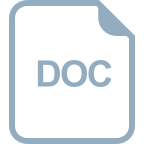