BP神经网络拟合激光中心直线函数代码
时间: 2023-10-10 17:14:18 浏览: 185
以下是使用Python编写的BP神经网络拟合激光中心直线函数的代码:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成数据
x = np.linspace(-10, 10, 1000)
y = 2*x + 1 + np.random.normal(0, 1, 1000)
# 构建BP神经网络
input_layer_size = 1 # 输入层节点数
hidden_layer_size = 10 # 隐层节点数
output_layer_size = 1 # 输出层节点数
learning_rate = 0.01 # 学习率
# 初始化权重和偏置
theta1 = np.random.randn(input_layer_size, hidden_layer_size)
bias1 = np.random.randn(hidden_layer_size)
theta2 = np.random.randn(hidden_layer_size, output_layer_size)
bias2 = np.random.randn(output_layer_size)
# 定义sigmoid函数
def sigmoid(z):
return 1 / (1 + np.exp(-z))
# 定义代价函数
def cost_function(y, y_pred):
return np.mean((y - y_pred)**2)
# 训练神经网络
for i in range(10000):
# 前向传播
z2 = np.dot(x, theta1) + bias1
a2 = sigmoid(z2)
y_pred = np.dot(a2, theta2) + bias2
# 计算代价
cost = cost_function(y, y_pred)
# 反向传播
delta3 = y_pred - y
delta2 = np.dot(delta3, theta2.T) * sigmoid(z2) * (1 - sigmoid(z2))
# 更新权重和偏置
theta2 -= learning_rate * np.dot(a2.T, delta3)
bias2 -= learning_rate * np.mean(delta3, axis=0)
theta1 -= learning_rate * np.dot(x.reshape(-1, 1), delta2)
bias1 -= learning_rate * np.mean(delta2, axis=0)
if i % 1000 == 0:
print("Iteration {}, cost {:.4f}".format(i, cost))
# 预测
y_pred = np.dot(sigmoid(np.dot(x, theta1) + bias1), theta2) + bias2
# 绘制拟合结果
plt.scatter(x, y)
plt.plot(x, y_pred, color="r")
plt.show()
```
该代码生成1000个随机样本,其中$x$和$y$之间的关系是$y=2x+1$,并添加了一些高斯噪声。然后,使用BP神经网络拟合这些数据,其中输入层只有一个节点,输出层也只有一个节点,隐层有10个节点。训练完成后,对于给定的$x$,可以预测相应的$y$。最后,使用Matplotlib绘制原始数据和拟合结果。
阅读全文
相关推荐
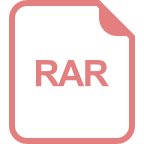
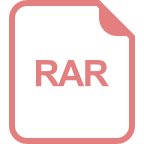
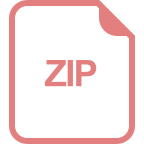
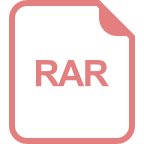
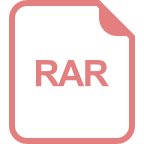
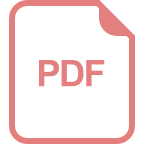
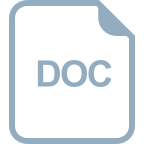
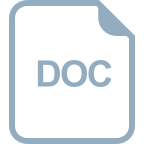