scala中val与var格式上的区别
时间: 2024-05-13 11:13:57 浏览: 117
在 Scala 中,`val` 和 `var` 都用于定义变量,但它们在格式上有区别:
- `val` 是不可变变量(immutable variable),用于定义一次赋值且不可更改的变量。
- `var` 是可变变量(mutable variable),用于定义可多次赋值的变量。
例如:
```scala
val x = 1 // 定义一个不可变变量 x,赋值为 1
x = 2 // 编译错误:reassignment to val
```
```scala
var y = 1 // 定义一个可变变量 y,赋值为 1
y = 2 // y 的值被修改为 2
```
在使用变量时,建议优先使用 `val`,因为它能够避免一些错误和副作用,例如多线程并发操作时的数据竞争问题。只有在必要的情况下才使用 `var`。
相关问题
如何用scala计算股票的Var
VaR(Value at Risk)是用于度量金融市场风险的一种方法,通常用于衡量投资组合可能面临的最大损失。在计算股票的VaR时,需要考虑股票的历史价格数据、波动率和投资组合的持仓情况等因素。以下是一种用Scala计算股票VaR的方法:
1. 收集股票历史价格数据,通常可以从金融数据提供商或交易所获取。
2. 计算股票收益率的均值和标准差,这是计算VaR所需的基本数据。假设有一个包含$n$个历史价格数据的向量$P=(p_1,p_2,...,p_n)$,则收益率向量为$R=(r_1,r_2,...,r_{n-1})$,其中$r_i=(p_{i+1}-p_i)/p_i$。
3. 使用收益率的均值和标准差来估计股票的未来风险。标准差可以使用Scala中的内置函数计算:`val sd = R.stdev()`
4. 计算VaR。假设投资组合价值为$V$,置信水平为$p$,则VaR可以使用以下公式计算:$VaR=V * z_p*sd$,其中$z_p$是正态分布的$p$分位数。在Scala中,可以使用Apache Commons Math库中的StatUtils类来计算正态分布的分位数:`val zp = StatUtils.inverseCumulativeProbability(StandardNormalDistribution(), p)`
5. 根据计算结果,可以得出投资组合可能面临的最大损失。例如,如果VaR为10000美元,那么在给定的置信水平下,投资组合可能面临最多10000美元的损失。
需要注意的是,VaR只是一种估计风险的方法,它并不能完全预测未来的风险。在实际投资中,投资者还应该考虑其他因素,如流动性风险、市场风险和操作风险等。
使用Scala 优化以下代码 def copyDirectory(source: File, destination: File): Unit = { if (source.isDirectory) { if (!destination.exists()) { destination.mkdir() } val files = source.listFiles() for (f <- files) { val newFile = new File(destination.getAbsolutePath + File.separator + f.getName) copyDirectory(f, newFile) } } else { val in = new FileInputStream(source) val out = new FileOutputStream(destination) val buffer = new Array[Byte](1024) var length = in.read(buffer) while (length > 0) { out.write(buffer, 0, length) length = in.read(buffer) } in.close() out.close() } }
以下是对 `copyDirectory` 方法进行优化的示例代码:
```scala
import java.io._
object FileCopy {
def main(args: Array[String]): Unit = {
val source = new File("path/to/source/directory")
val destination = new File("path/to/destination/directory")
copyDirectory(source, destination)
}
def copyDirectory(source: File, destination: File): Unit = {
if (!source.exists()) {
return
}
if (source.isDirectory) {
if (!destination.exists()) {
destination.mkdir()
}
val files = Option(source.listFiles()).getOrElse(Array.empty[File])
for (f <- files) {
val newFile = new File(destination, f.getName)
copyDirectory(f, newFile)
}
} else {
val in = new FileInputStream(source)
val out = new FileOutputStream(destination)
try {
val buffer = new Array[Byte](4096)
var bytesRead = in.read(buffer)
while (bytesRead != -1) {
out.write(buffer, 0, bytesRead)
bytesRead = in.read(buffer)
}
} finally {
in.close()
out.close()
}
}
}
}
```
在上述代码中,我们首先添加了一个条件判断,如果源文件或目录不存在,则直接返回。接着,我们使用 `Option` 对 `source.listFiles()` 方法的返回值进行了安全处理,如果为空则返回一个空数组。这样可以避免在遍历文件时出现空指针异常。另外,我们使用了 `File` 的构造函数来创建新文件对象,而不是使用字符串拼接的方式。这样可以提高代码的可读性。
在文件复制的部分,我们使用了 try-finally 语句块来确保输入和输出流都能被正确地关闭。此外,我们将缓冲区的大小增加到了 4096 字节,这样可以提高文件复制的效率。
阅读全文
相关推荐
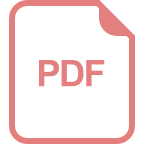
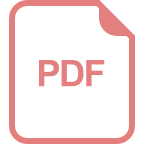
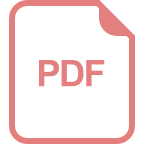
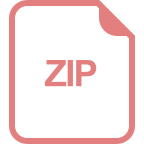
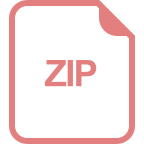
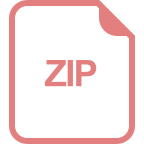
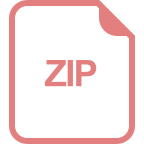
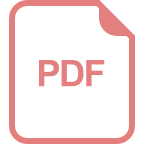
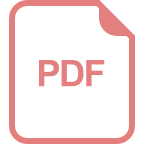
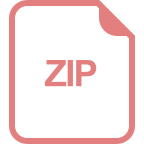
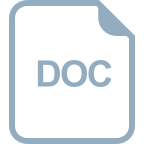
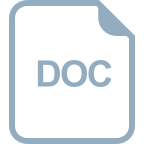
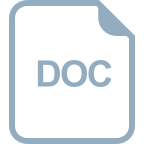
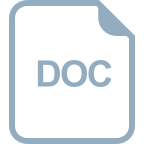
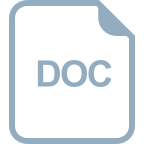
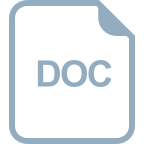
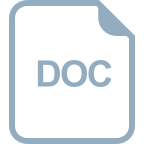