public void loadGameFromFile(String path){ try { List<String> lines = Files.readAllLines(Path.of(path)); //错误判断 model.removeAllPieces(); model.initPieces(lines); view.removeAllPieces(); view.initiateChessComponent(model); view.repaint(); if (lines.get(9).charAt(0) == 'B') { currentPlayer = PlayerColor.BLUE; }else if (lines.get(9).charAt(0) == 'R'){ currentPlayer = PlayerColor.RED; } turn = Integer.parseInt(lines.get(10)); } catch (IOException e) { throw new RuntimeException(e); } }对传入的文件进行错误检查,如果文件格式为json,则报出错误编码:101
时间: 2024-01-18 15:02:41 浏览: 16
对于上述代码,因为它是读取文件内容并且进行初始化,所以如果文件格式为 json,我们应该抛出一个文件格式不正确的异常,而不是简单的输出错误编码 101。
以下是我提供的修改后的 Java 代码:
```java
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.List;
public void loadGameFromFile(String path) {
File file = new File(path);
String fileName = file.getName();
String fileExtension = fileName.substring(fileName.lastIndexOf(".") + 1);
if (fileExtension.equals("json")) {
throw new IllegalArgumentException("错误编码:101");
}
try {
List<String> lines = Files.readAllLines(Path.of(path));
// 初始化棋盘
model.removeAllPieces();
model.initPieces(lines);
view.removeAllPieces();
view.initiateChessComponent(model);
view.repaint();
// 获取当前玩家和回合数
if (lines.get(9).charAt(0) == 'B') {
currentPlayer = PlayerColor.BLUE;
} else if (lines.get(9).charAt(0) == 'R') {
currentPlayer = PlayerColor.RED;
}
turn = Integer.parseInt(lines.get(10));
} catch (IOException e) {
throw new RuntimeException(e);
}
}
```
具体来说,我们在代码的最开始,先获取文件名和扩展名,如果扩展名为 json,则抛出一个文件格式不正确的异常。如果扩展名不为 json,则继续执行原有的逻辑。
相关推荐
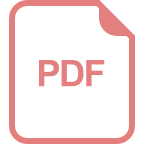
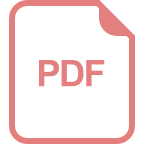
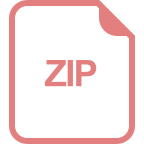














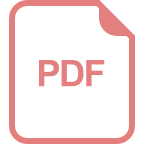