java怎么实现数组螺旋排序
时间: 2024-10-10 13:07:05 浏览: 37
在Java中实现数组的螺旋排序(也称为矩阵螺旋遍历),它不是标准的排序算法,而是一种数据访问和遍历的方式。这种排序实际上并不是对数组元素进行线性排列,而是按照螺旋的形式从左上角开始,沿着顺时针方向逐层移动到右下角。由于题目并未直接涉及排序操作,这里我们将描述如何通过二维数组的螺旋遍历来展示元素,而不是排序。
首先,创建一个二维数组并填充数据,然后编写一个函数来按照螺旋顺序打印数组:
```java
public void spiralPrint(int[][] matrix) {
if (matrix == null || matrix.length == 0)
return;
int m = matrix.length, n = matrix[0].length;
int startRow = 0, endRow = m - 1;
int startCol = 0, endCol = n - 1;
while (startRow <= endRow && startCol <= endCol) {
// Print top row
for (int i = startCol; i <= endCol; i++) {
System.out.print(matrix[startRow][i] + " ");
}
startRow++;
// Print right column
for (int i = startRow; i <= endRow; i++) {
System.out.print(matrix[i][endCol] + " ");
}
endCol--;
// Print bottom row
if (startRow <= endRow) {
for (int i = endCol; i >= startCol; i--) {
System.out.print(matrix[endRow][i] + " ");
}
endRow--;
}
// Print left column
if (startCol <= endCol) {
for (int i = endRow; i >= startRow; i--) {
System.out.print(matrix[i][startCol] + " ");
}
startCol++;
}
}
}
```
在这个例子中,`spiralPrint`函数会按照螺旋路径依次访问数组中的每个元素。如果你想在访问后更新数组元素,那么这就不算螺旋排序,因为它并没有改变元素的原始顺序。
阅读全文
相关推荐
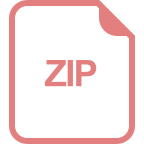
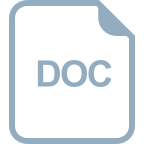
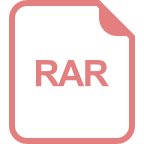
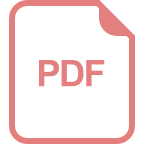
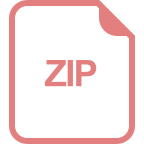
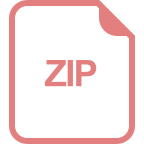
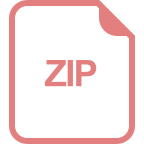
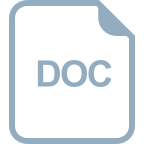
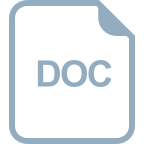
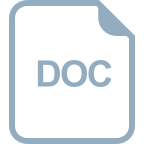
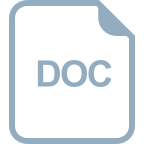
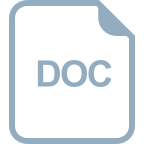
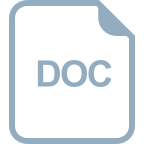
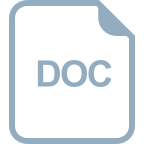
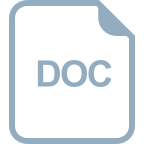
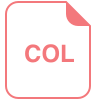
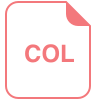
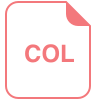
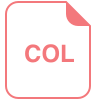