vue marquee 数据改变,文字没有重新渲染
时间: 2024-03-13 15:18:01 浏览: 11
如果您使用的是 Vue Marquee 组件,并且在数据改变时文字没有重新渲染,那么可能是因为您没有正确地使用组件的 props。
请确保您已经将需要滚动的文字传递给了组件的 `text` prop,并且在数据改变时更新了该 prop 的值。例如:
```html
<vue-marquee :text="scrollingText"></vue-marquee>
```
然后在您的 Vue 实例中,更新 `scrollingText` 的值即可触发文字重新渲染:
```js
export default {
data() {
return {
scrollingText: '这是需要滚动的文本'
}
},
methods: {
updateText() {
this.scrollingText = '更新后的文本'
}
}
}
```
如果您已经正确地使用了组件的 props,但仍然遇到问题,请检查您的浏览器控制台是否有任何错误或警告信息,并确保您使用的是最新版本的 Vue Marquee 组件。
相关问题
vue表格数据改变页面实时渲染
Vue是一款现代化的JavaScript框架,通过数据绑定和虚拟DOM的概念,可以实现实时渲染页面。在Vue中,表格数据的改变可以很方便地触发页面的实时渲染。
首先,我们可以利用Vue的数据绑定功能来实现表格数据的实时渲染。Vue的数据绑定可以将数据和模板进行绑定,在数据发生改变时,Vue会自动更新相关的DOM元素。例如,当表格中的数据发生改变时,我们可以使用Vue的数据绑定将数据与表格模板进行绑定,使得数据的改变可以实时响应到表格上。
另外,Vue的虚拟DOM机制也能够保证页面的实时渲染。Vue会根据数据的变化,生成一颗虚拟DOM树,然后通过比较新旧虚拟DOM树的差异,最终只更新需要改变的部分,而不是整个页面。这样就能够大大提高页面的渲染效率,实现实时渲染。
除了数据绑定和虚拟DOM,Vue还提供了一些其他的功能来实现实时渲染。例如,计算属性和侦听器可以用来监测数据的变化并在变化时执行相应的操作;watch深度观察可以监听数组和对象的变化;过滤器可以对数据进行格式化等。
总而言之,通过Vue的数据绑定、虚拟DOM以及其他相关功能,我们可以很方便地实现表格数据的实时渲染。在表格数据发生改变时,Vue会自动更新相关的DOM元素,保证页面能够实时呈现最新的数据。这也是Vue成为流行的JavaScript框架之一的原因之一。
vue使页面数据不变,重新渲染
在 Vue 中,如果要重新渲染页面而不改变数据,可以使用 `key` 属性来强制重新渲染组件。当 `key` 值发生变化时,Vue 会强制重新渲染组件,即使数据没有发生变化。
例如,可以在需要重新渲染的组件上加上 `key` 属性,将其设置为一个动态变量,如下所示:
```html
<template>
<div :key="componentKey">
<!-- 组件内容 -->
</div>
</template>
<script>
export default {
data() {
return {
componentKey: 0
};
},
methods: {
reRender() {
this.componentKey += 1;
}
}
}
</script>
```
当需要重新渲染组件时,只需要调用 `reRender` 方法,就会强制重新渲染组件。需要注意的是,重新渲染组件可能会影响性能,因此应该谨慎使用。
相关推荐
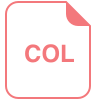
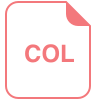









