某班的一次考试所有学生的考试成绩都记录在一个文件in.data之中,文件内容格式如下: 张三的成绩是72分,李四成绩是69分,刘小林的成绩是95分。 现在需要对该文件进行处理,将其内容拷贝到另一个目标文件out.data之中,并统计所有学生成绩平均分(保留2为小数)。 请编码实现该成绩统计系统。
时间: 2024-02-09 22:09:15 浏览: 102
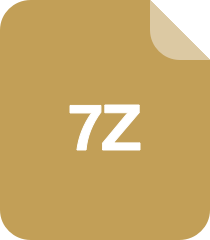
利用实现数据库实现学生分班系统源码

好的,让我们来编写代码实现这个需求。以下是完整的 Java 代码:
```java
import java.io.*;
import java.util.Scanner;
public class ScoreAnalyzer {
public static final String SRC_FILE = ScoreAnalyzer.class.getClassLoader().getResource("").getFile() + "in.data"; // 源数据文件名
public static final String RLT_FILE = ScoreAnalyzer.class.getClassLoader().getResource("").getFile() + "out.data"; // 最终结果存放文件名
public static void main(String[] args) {
File inFile = new File(SRC_FILE);
File outFile = new File(RLT_FILE);
try (BufferedInputStream bis = new BufferedInputStream(new FileInputStream(inFile));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream(outFile));
Scanner scanner = new Scanner(bis)) {
byte[] buffer = new byte[1024];
int len;
while ((len = bis.read(buffer)) != -1) {
bos.write(buffer, 0, len);
}
System.out.println("文件拷贝完成!");
double sum = 0;
int count = 0;
scanner.useDelimiter("[^0123456789.]+");
while (scanner.hasNextDouble()) {
double score = scanner.nextDouble();
sum += score;
count++;
}
double avgScore = count == 0 ? 0 : sum / count;
System.out.printf("所有学生的平均分为:%.2f\n", avgScore);
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上代码中,我们使用 BufferedInputStream 和 BufferedOutputStream 对 FileInputStream 和 FileOutputStream 进行包装,以提高读写的效率。在文件拷贝过程中,我们使用 byte 数组缓存读取的数据,并使用 while 循环读取输入文件中的数据到 byte 数组中,并将每次读取的数据通过 BufferedOutputStream 对象写入到目标文件中。在文件分析过程中,我们使用 try-with-resources 语句块确保 Scanner 对象的正确关闭,并使用 scanner.useDelimiter("[^0123456789.]+") 设置非数字类型作为分隔符,对应的正则表达式为"[^0123456789.]+"。然后使用 hasNextDouble() 和 nextDouble() 方法获取分割出的每一项成绩,统计总成绩和项数,计算平均值。注意除数不能为0的情况。最后使用 System.out.printf() 方法输出结果,保留2位小数。
阅读全文
相关推荐
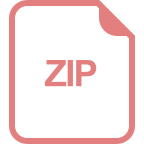

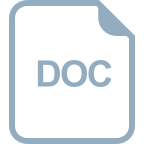
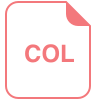
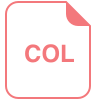
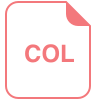
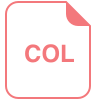
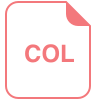
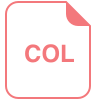
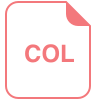
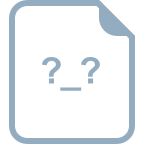
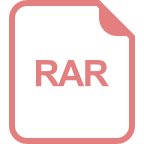
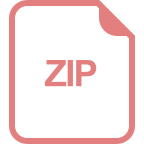
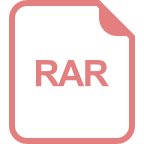