自定义QTreeview和QFileSystemModel,实现时在单独的线程使用定时器每隔500毫秒扫描100文件逐步构建整个模型,并显示在自定义QTreeview上,使用C++给出完整代码
时间: 2023-11-22 12:53:49 浏览: 36
以下是使用定时器和单独线程构建QFileSystemModel并显示在自定义QTreeView上的C++代码示例:
```
#include <QtWidgets>
#include <QFileSystemModel>
class CustomTreeView : public QTreeView
{
Q_OBJECT
public:
CustomTreeView(QWidget *parent = nullptr) : QTreeView(parent) {}
~CustomTreeView() {}
void setModel(QAbstractItemModel *model) override
{
QTreeView::setModel(model);
expandAll();
}
};
class FileSystemModelThread : public QThread
{
Q_OBJECT
public:
FileSystemModelThread(QObject *parent = nullptr)
: QThread(parent), m_model(nullptr) {}
~FileSystemModelThread() {}
void setRootPath(const QString &path)
{
m_rootPath = path;
}
QFileSystemModel* model()
{
return m_model;
}
signals:
void modelReady(QAbstractItemModel *model);
protected:
void run() override
{
m_model = new QFileSystemModel();
m_model->setRootPath(m_rootPath);
QTimer timer;
timer.setInterval(500);
int row = 0;
QModelIndex parentIndex = QModelIndex();
while (m_model->canFetchMore(parentIndex))
{
m_model->fetchMore(parentIndex);
row += 100;
QModelIndex index = m_model->index(row, 0, parentIndex);
if (!index.isValid())
{
continue;
}
CustomTreeView *treeView = qobject_cast<CustomTreeView*>(parent());
if (treeView)
{
QAbstractItemModel *model = m_model->takeColumn(0, parentIndex);
emit modelReady(model);
}
QEventLoop loop;
connect(&timer, &QTimer::timeout, &loop, &QEventLoop::quit);
timer.start();
loop.exec();
}
}
private:
QString m_rootPath;
QFileSystemModel *m_model;
};
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr)
: QMainWindow(parent), m_fileSystemModelThread(nullptr)
{
QWidget *centralWidget = new QWidget(this);
QVBoxLayout *layout = new QVBoxLayout(centralWidget);
setCentralWidget(centralWidget);
m_treeView = new CustomTreeView(centralWidget);
layout->addWidget(m_treeView);
QPushButton *button = new QPushButton("Start", centralWidget);
layout->addWidget(button);
connect(button, &QPushButton::clicked, this, &MainWindow::startFileSystemModelThread);
}
~MainWindow() {}
private slots:
void modelReady(QAbstractItemModel *model)
{
m_treeView->setModel(model);
}
private:
void startFileSystemModelThread()
{
if (m_fileSystemModelThread)
{
return;
}
m_fileSystemModelThread = new FileSystemModelThread(this);
m_fileSystemModelThread->setRootPath(QDir::currentPath());
connect(m_fileSystemModelThread, &FileSystemModelThread::modelReady, this, &MainWindow::modelReady);
m_fileSystemModelThread->start();
}
private:
CustomTreeView *m_treeView;
FileSystemModelThread *m_fileSystemModelThread;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
#include "main.moc"
```
在这个示例中,我们创建了一个CustomTreeView类,用于设置模型并展开所有节点。我们还创建了一个FileSystemModelThread类,用于在单独的线程中构建QFileSystemModel,并使用定时器每隔500毫秒扫描100个文件。在构建每个文件夹的子项后,我们将模型发送到MainWindow类的槽中,该槽将模型设置为CustomTreeView的模型。最后,我们在MainWindow类中创建了一个QPushButton,用于启动FileSystemModelThread类的实例。
请注意,此代码示例仅用于演示目的,实际生产代码需要处理线程和模型的更多细节,例如取消线程和处理模型变化的方式。
相关推荐
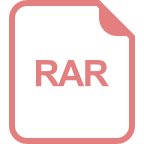














