java计算器程序代码在软件中运行
时间: 2024-03-07 10:48:51 浏览: 61
以下是一个示例Java计算器程序的代码,您可以将其复制并在Java开发环境中运行:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class Calculator extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JPanel panel;
private JTextField display;
private JButton[] buttons;
private String[] labels = { "Backspace", "", "", "CE", "C", "7", "8", "9", "/", "sqrt", "4", "5", "6",
"*", "%", "1", "2", "3", "-", "1/x", "0", "+/-", ".", "+", "=" };
private double result = 0;
private String operator = "=";
private boolean startOfNumber = true;
public Calculator() {
display = new JTextField(35);
panel = new JPanel();
display.setText("0.0");
panel.setLayout(new GridLayout(0, 5, 3, 3));
buttons = new JButton[25];
int index = 0;
for (int rows = 0; rows < 5; rows++) {
for (int cols = 0; cols < 5; cols++) {
buttons[index] = new JButton(labels[index]);
buttons[index].addActionListener(this);
panel.add(buttons[index]);
index++;
}
}
add(display, BorderLayout.NORTH);
add(panel, BorderLayout.CENTER);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
pack();
setVisible(true);
}
public void actionPerformed(ActionEvent event) {
String command = event.getActionCommand();
if (command.charAt(0) == 'C') {
startOfNumber = true;
result = 0;
operator = "=";
display.setText("0.0");
} else if (command.charAt(0) >= '0' && command.charAt(0) <= '9' || command.equals(".")) {
if (startOfNumber == true) {
display.setText(command);
} else {
display.setText(display.getText() + command);
}
startOfNumber = false;
} else {
if (startOfNumber) {
if (command.equals("-")) {
display.setText(command);
startOfNumber = false;
} else {
operator = command;
}
} else {
double x = Double.parseDouble(display.getText());
calculate(x);
operator = command;
startOfNumber = true;
}
}
}
private void calculate(double n) {
if (operator.equals("+")) {
result += n;
} else if (operator.equals("-")) {
result -= n;
} else if (operator.equals("*")) {
result *= n;
} else if (operator.equals("/")) {
try {
result /= n;
} catch (ArithmeticException e) {
display.setText("Error");
}
} else if (operator.equals("%")) {
result %= n;
} else if (operator.equals("=")) {
result = n;
} else if (operator.equals("sqrt")) {
result = Math.sqrt(n);
} else if (operator.equals("1/x")) {
try {
result = 1 / n;
} catch (ArithmeticException e) {
display.setText("Error");
}
} else if (operator.equals("+/-")) {
result = -n;
}
display.setText("" + result);
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
}
}
```
请注意,此代码使用Java Swing库创建了一个GUI界面,其中包含数字、操作符和其他控件。 actionPerformed方法是一个事件处理程序,用于处理用户单击按钮时发生的事件。在此方法中,我们执行所选操作并更新显示结果。您可以将此代码复制并在Java开发环境中编译和运行。
阅读全文
相关推荐
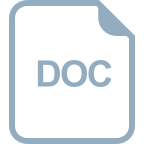
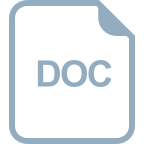
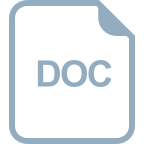
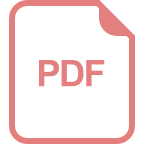
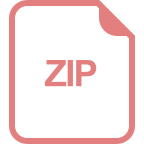
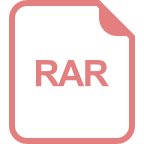
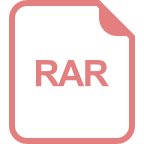
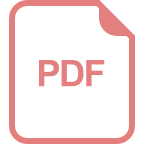
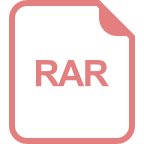
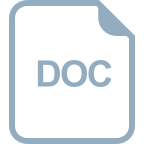
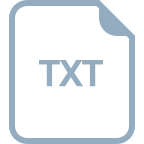
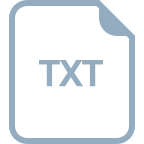
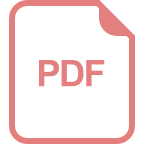
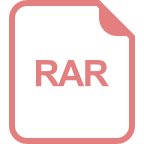
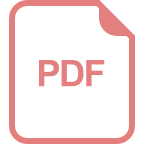
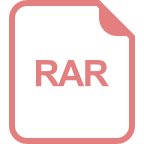
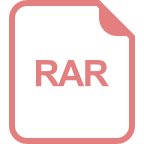
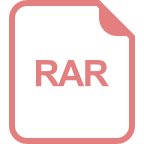