通过Windows的记事本,将如下内容,复制并保存到:word.txt,文件可以存储在任意位置。 python java c++ python is the best language in the world do you love python the course name is python programming 通过文件读取操作,读取此文件,统计python单词出现的次数。 提示:使用两种方法。 方法1:读取全部内容,通过字符串count方法统计python单词数量 方法2:读取内容,一行一行读取,使用strip()方法去掉开头和结尾及换行符。
时间: 2023-11-27 17:50:35 浏览: 15
方法1:
```python
with open('word.txt', 'r') as file:
content = file.read()
count = content.count('python')
print(f"Python单词出现了{count}次")
```
方法2:
```python
with open('word.txt', 'r') as file:
count = 0
for line in file:
line = line.strip()
count += line.count('python')
print(f"Python单词出现了{count}次")
```
相关问题
python 打开 Windows 的记事本软件,复制下面的文字内容,保存文件并命名为“in.txt”。 in.txt hello world a program An apple hello C++ A man 键盘输入正整数n,从文件"in.txt"中读入n行,将其中以字母A开头的行打印到屏幕上。 输入格式: 从键盘输入一个正整数n。 输出格式: 输出前n行中所有以字母A开头的行。若未找到符合条件的行,则输出"not found";若输入的n超出了文件的总行数,则输出"illegal input"。 输入样例: 5 2 6 输出样例: An apple A man not found illegal input
好的,根据您的要求,我提供以下 Python 代码实现:
```
n = int(input("请输入正整数n:"))
try:
with open("in.txt") as f:
lines = f.readlines()
if n > len(lines):
print("illegal input")
else:
found = False
for line in lines[:n]:
if line.startswith("A"):
print(line.strip())
found = True
if not found:
print("not found")
except FileNotFoundError:
print("文件不存在")
```
您可以将以上代码保存在一个 Python 文件中,然后运行该文件,根据提示输入正整数 n 即可。注意,该文件需要与 in.txt 文件在同一目录下。
C++实现用记事本产生一个文本文件data.txt,其内容为若干实数,数据之间以空白字符分割。编程从该文件中读入这些实数,求出这些实数的平均值,在程序中创建并产生一个文本文件result.txt,内容为data.txt中的全体实数,每行5个数,最后一行是求出的平均值。
好的,我来为你提供一段C++代码来实现你的需求。
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <iomanip>
using namespace std;
int main()
{
// 打开 data.txt 文件并读取实数
ifstream fin("data.txt");
double num;
vector<double> numbers;
while (fin >> num)
{
numbers.push_back(num);
}
fin.close();
// 计算实数的平均值
double sum = 0;
for (auto n : numbers)
{
sum += n;
}
double average = sum / numbers.size();
// 将实数写入 result.txt 文件
ofstream fout("result.txt");
int count = 0;
for (auto n : numbers)
{
fout << fixed << setprecision(2) << n << " ";
count++;
if (count % 5 == 0)
{
fout << endl;
}
}
// 写入平均值
fout << endl << "Average: " << fixed << setprecision(2) << average;
fout.close();
return 0;
}
```
这段代码与之前Python代码的思路类似,主要分为以下几个步骤:
1. 打开 data.txt 文件并读取其中的实数,存储在一个vector `numbers` 中。
2. 计算 `numbers` 中所有实数的平均值,存储在变量 `average` 中。
3. 打开 result.txt 文件并将 `numbers` 中的实数写入文件,每行最多写入 5 个数。
4. 在最后一行写入平均值。
希望这个C++代码能够帮到你,如有其他问题,欢迎随时提出。
阅读全文
相关推荐
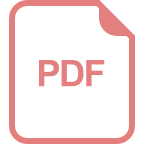
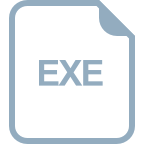
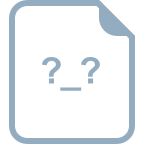
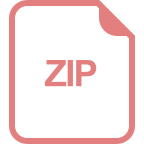
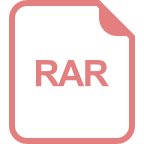
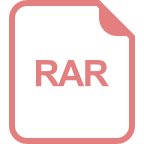
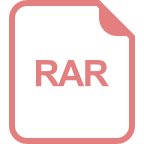
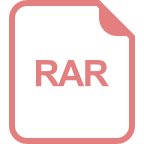
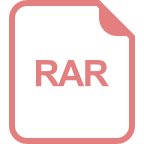
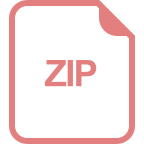
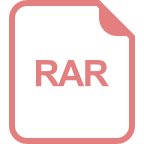
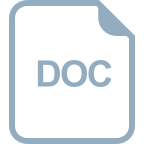
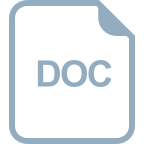
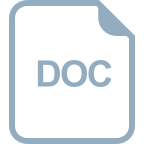
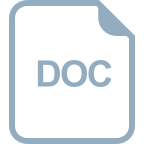