使用flask框架,使用HTML语言,调用HTML文件创建templates文件夹可以是其他名称吗
时间: 2023-12-10 19:05:33 浏览: 79
在 Flask 中,可以通过配置 `app = Flask(__name__, template_folder='my_templates')` 来指定模板文件的存放路径。其中,`template_folder` 参数表示模板文件所在的文件夹路径,你可以将其设置为任何你喜欢的名称。
比如,你可以这样创建一个名为 `my_templates` 的文件夹,并将其作为模板文件的存放路径:
```python
from flask import Flask, render_template
app = Flask(__name__, template_folder='my_templates')
@app.route('/')
def index():
return render_template('index.html')
if __name__ == '__main__':
app.run()
```
在上述代码中,`template_folder` 被设置为 `'my_templates'`,表示模板文件将会存放在 `my_templates` 文件夹中。当你调用 `render_template('index.html')` 时,Flask 就会在 `my_templates` 文件夹中查找名为 `index.html` 的模板文件。
相关问题
Flask框架渲染html方法
在 Flask 框架中,可以使用 Jinja2 模板引擎来渲染 HTML 模板,具体方法如下:
1. 在 Flask 应用程序的根目录下创建 templates 文件夹,并在该文件夹中创建 HTML 模板文件。
2. 在 Flask 应用程序中导入 render_template 函数。
```python
from flask import Flask, render_template
```
3. 在视图函数中调用 render_template 函数,并将 HTML 模板文件名作为参数传递给该函数。
```python
@app.route('/')
def index():
return render_template('index.html')
```
其中,index.html 是在 templates 文件夹中创建的 HTML 模板文件的文件名。
4. 在 HTML 模板文件中使用 Jinja2 语法来渲染动态内容。
例如,在 Flask 应用程序中定义一个名为 name 的变量,并将其传递给 render_template 函数:
```python
@app.route('/')
def index():
name = 'John'
return render_template('index.html', name=name)
```
在 HTML 模板文件中,可以使用 {{ 变量名 }} 的语法来引用该变量:
```html
<!DOCTYPE html>
<html>
<head>
<title>Hello, {{ name }}!</title>
</head>
<body>
<h1>Hello, {{ name }}!</h1>
</body>
</html>
```
在浏览器中访问该 Flask 应用程序时,将会显示一个包含动态内容的 HTML 页面。
python flask 调用html模板
可以使用Flask框架中的render_template()函数来调用HTML模板。具体步骤如下:
1. 在Flask应用程序中导入render_template()函数:
```
from flask import Flask, render_template
```
2. 在应用程序中创建一个路由,使用render_template()函数来渲染HTML模板:
```
@app.route('/hello')
def hello():
return render_template('hello.html')
```
在上面的例子中,/hello是一个路由,当用户访问该路由时,会调用hello()函数,并返回渲染后的hello.html模板。
3. 在Flask应用程序中创建一个templates文件夹,将HTML模板放在该文件夹中。
注意:Flask框架默认会在应用程序根目录下的templates文件夹中查找HTML模板。
示例代码:
```
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/hello')
def hello():
return render_template('hello.html')
if __name__ == '__main__':
app.run(debug=True)
```
在上面的代码中,我们创建了一个Flask应用程序,并创建了一个/hello路由,当用户访问该路由时,会调用hello()函数,并返回渲染后的hello.html模板。如果需要在开发环境下调试应用程序,可以将debug参数设置为True,这样就可以看到详细的调试信息。
阅读全文
相关推荐
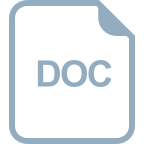
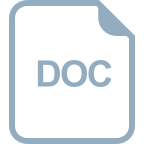
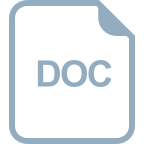
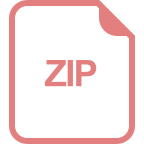
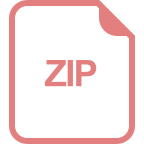
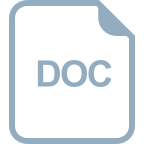
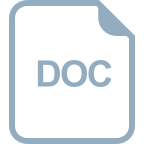
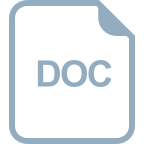
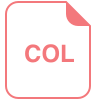
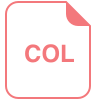
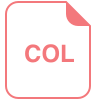
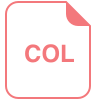
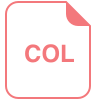



