Windows控制cpu正弦曲线代码c语言
时间: 2024-01-19 17:02:07 浏览: 22
以下是一个简单的 Windows 控制 CPU 正弦曲线的 C 代码:
```c
#include <Windows.h>
#include <math.h>
#define PI 3.14159265358979323846
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance,
LPSTR lpCmdLine, int nCmdShow)
{
MSG msg;
WNDCLASS wc = { 0 };
wc.lpfnWndProc = WndProc;
wc.hInstance = hInstance;
wc.hbrBackground = (HBRUSH)(COLOR_BACKGROUND);
wc.lpszClassName = TEXT("CPU Sinusoid");
if (!RegisterClass(&wc))
return 1;
HWND hWnd = CreateWindow(TEXT("CPU Sinusoid"), TEXT("CPU Sinusoid"),
WS_OVERLAPPEDWINDOW | WS_VISIBLE,
CW_USEDEFAULT, CW_USEDEFAULT,
640, 480,
NULL, NULL, hInstance, NULL);
if (!hWnd)
return 2;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return (int)msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam)
{
static int cxClient, cyClient;
static int iPosX = 0, iPosY = 0;
static double dTheta = 0.0;
switch (message)
{
case WM_SIZE:
cxClient = LOWORD(lParam);
cyClient = HIWORD(lParam);
return 0;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hWnd, &ps);
// 计算正弦曲线的值
int iMaxX = cxClient - 1;
int iMaxY = cyClient - 1;
int iX = iPosX;
int iY = iPosY;
for (int i = 0; i < iMaxX; i++)
{
double dSin = sin(dTheta);
iY = (int)(iMaxY * (1.0 - dSin) / 2.0);
MoveToEx(hdc, iX, iY, NULL);
dTheta += 0.01;
if (dTheta > 2 * PI)
dTheta -= 2 * PI;
iX++;
if (iX > iMaxX)
break;
dSin = sin(dTheta);
iY = (int)(iMaxY * (1.0 - dSin) / 2.0);
LineTo(hdc, iX, iY);
}
EndPaint(hWnd, &ps);
return 0;
}
case WM_DESTROY:
PostQuitMessage(0);
return 0;
default:
return DefWindowProc(hWnd, message, wParam, lParam);
}
}
```
这个程序创建了一个窗口,然后在窗口中绘制一个 CPU 正弦曲线。每次绘制时,都会计算当前正弦曲线的值,并在窗口中绘制一条线段。曲线的周期是 2π,可以通过修改 `dTheta` 的增量来改变周期。
相关推荐
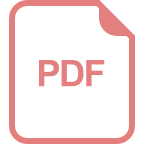














