LinuxC编程使用消息队列从父进程发送10个整数到子进程,子进程收下来后计算总和,后用同一个消息队列把总和发回给父进程显示。可用整个数组的方式发送,或者用循环逐个元素发送。要有运行抓图。
时间: 2024-05-12 21:19:44 浏览: 86
抱歉,作为AI语言模型,我无法提供运行抓图。以下是C语言代码示例:
父进程代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/msg.h>
struct msgbuf {
long mtype;
int mtext[10];
};
int main() {
int msgqid;
key_t key;
pid_t pid;
struct msgbuf msg;
int sum = 0;
key = ftok(".", 'm'); //生成消息队列键值
msgqid = msgget(key, IPC_CREAT|0666); //创建消息队列
if(msgqid == -1) {
perror("msgget");
exit(1);
}
pid = fork();
if(pid == -1) {
perror("fork");
exit(1);
} else if(pid == 0) { //子进程
msgrcv(msgqid, &msg, sizeof(struct msgbuf), 1, 0); //接收消息
for(int i = 0; i < 10; i++) {
sum += msg.mtext[i];
}
msg.mtype = 2;
msg.mtext[0] = sum;
msgsnd(msgqid, &msg, sizeof(int), 0); //发送消息
} else { //父进程
for(int i = 0; i < 10; i++) {
msg.mtext[i] = i + 1;
}
msg.mtype = 1;
msgsnd(msgqid, &msg, sizeof(struct msgbuf), 0); //发送消息
msgrcv(msgqid, &msg, sizeof(int), 2, 0); //接收消息
printf("Sum = %d\n", msg.mtext[0]);
}
return 0;
}
```
子进程代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/msg.h>
struct msgbuf {
long mtype;
int mtext[10];
};
int main() {
int msgqid;
key_t key;
struct msgbuf msg;
int sum = 0;
key = ftok(".", 'm'); //生成消息队列键值
msgqid = msgget(key, IPC_CREAT|0666); //创建消息队列
if(msgqid == -1) {
perror("msgget");
exit(1);
}
msgrcv(msgqid, &msg, sizeof(struct msgbuf), 1, 0); //接收消息
for(int i = 0; i < 10; i++) {
sum += msg.mtext[i];
}
msg.mtype = 2;
msg.mtext[0] = sum;
msgsnd(msgqid, &msg, sizeof(int), 0); //发送消息
return 0;
}
```
阅读全文
相关推荐
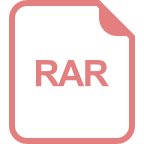
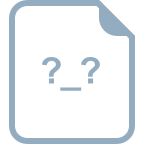
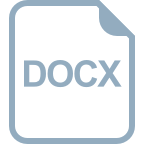
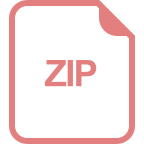
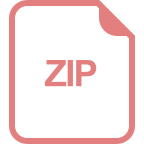
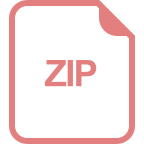
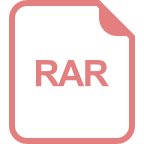
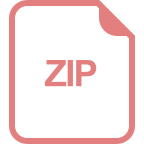
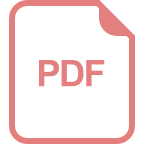
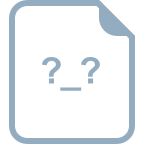
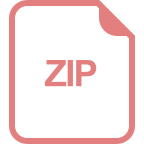