python 获取xml某个元素的属性
时间: 2024-02-27 19:51:51 浏览: 33
你可以使用Python的ElementTree库来获取XML中某个元素的属性。具体步骤如下:
1. 首先,使用ElementTree库中的parse()方法来解析XML文件,将其转换为一个Element对象。
```
import xml.etree.ElementTree as ET
tree = ET.parse('file.xml')
root = tree.getroot()
```
2. 然后,使用Element对象的attrib属性获取该元素的所有属性,并使用属性名获取特定属性的值。
```
# 获取元素属性
for elem in root.iter('element_name'):
attr_value = elem.attrib.get('attribute_name')
```
这样,你就可以获取XML文件中特定元素的属性值了。
相关问题
python自动抓取html元素
要自动抓取 HTML 元素,你可以使用 Python 中的 BeautifulSoup 库。这个库可以从 HTML 和 XML 文件中提取数据并解析文档,然后使用类似于树形结构的方法来遍历 HTML 元素。
以下是一个示例代码,演示如何使用 BeautifulSoup 库解析 HTML 并获取元素:
```python
from bs4 import BeautifulSoup
import requests
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 获取所有 a 标签
a_tags = soup.find_all('a')
for a in a_tags:
print(a.get('href'))
# 获取某个 div 标签中的所有 p 标签
div = soup.find('div', {'class': 'example'})
p_tags = div.find_all('p')
for p in p_tags:
print(p.text)
```
在这个示例中,我们首先获取了一个网站的 HTML 内容,然后使用 BeautifulSoup 库解析 HTML。我们接着获取了网站中的所有 a 标签和某个 div 标签中的所有 p 标签。最后我们打印出了每个 a 标签的 href 属性和每个 p 标签的文本内容。
Python xpath属性
在使用Python的XPath解析库(例如lxml)时,可以通过以下方式选择元素的属性:
- 选取特定属性的元素:`//tag[@attribute]`
- 选取特定属性值的元素:`//tag[@attribute='value']`
- 选取包含某个属性的元素:`//tag[@*]`
其中,`tag`为标签名,`attribute`为属性名,`value`为属性值。例如,要选取所有`<a>`标签中`href`属性为`"https://www.example.com"`的元素,可以使用如下XPath表达式:
```xpath
//a[@href="https://www.example.com"]
```
在Python中使用lxml库进行XPath解析时,可以通过`Element.xpath()`方法来选取元素。例如,选取上述XPath表达式所匹配的所有元素可以使用以下代码:
```python
from lxml import etree
html = """
<html>
<body>
<a href="https://www.example.com">Example</a>
<a href="https://www.google.com">Google</a>
<a href="https://www.example.com">Another Example</a>
</body>
</html>
"""
root = etree.HTML(html)
elements = root.xpath('//a[@href="https://www.example.com"]')
print(elements) # 输出两个<a>元素
```
输出结果为:
```
[<Element a at 0x7f9e8f426f48>, <Element a at 0x7f9e8f426f98>]
```
其中,`Element`对象代表一个XML元素,可以通过其`text`和`attrib`属性分别获取元素的文本和属性。
相关推荐
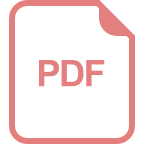









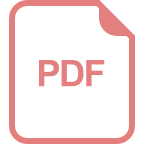
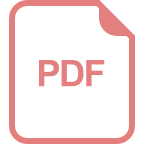
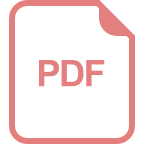
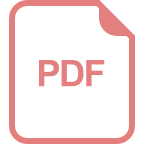