Create a progress meter a) Many GUI applications have metres that indicate the progress made so far towards completing an activity as a percentage of the anticipated total activity. Write a program to display a progress metre as follows. The metre consists of a window with a column of 11 labels. The first 10 labels indicate progress steps of 10% and are initially red. The bottom label shows the amount of activity remaining as a textual percentage, and is initially set to “0%”. (Correct layout: 0.25P, Class extends JFrame: 0.25P, Class follows OOP principles: 0.25P, Global set-up in main method: 0.25P)2 b) Create functionality to simulate steps of 10% progress. For this the user repeatedly presses return on the console inside Eclipse (use a Scanner object for this). Every time, the top-most red label is changed to green and the progress label value is incremented by 10 until that value is 100%. Make sure that no errors occur if the user tries to go past 100%.
时间: 2024-02-14 12:03:01 浏览: 36
Sure, I can help you with that! Here's an example code in Java that creates a progress meter:
```java
import javax.swing.*;
import java.awt.*;
public class ProgressMeter extends JFrame {
private JLabel[] steps = new JLabel[11];
private JLabel progressLabel;
private int progress = 0;
public ProgressMeter() {
setTitle("Progress Meter");
setSize(200, 300);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// Create step labels
for (int i = 0; i < steps.length - 1; i++) {
steps[i] = new JLabel((i + 1) * 10 + "%");
steps[i].setForeground(Color.RED);
steps[i].setFont(new Font("Arial", Font.PLAIN, 12));
steps[i].setHorizontalAlignment(SwingConstants.CENTER);
add(steps[i]);
}
// Create progress label
progressLabel = new JLabel("0%");
progressLabel.setForeground(Color.BLACK);
progressLabel.setFont(new Font("Arial", Font.PLAIN, 14));
progressLabel.setHorizontalAlignment(SwingConstants.CENTER);
add(progressLabel);
// Set layout
setLayout(new GridLayout(11, 1));
setVisible(true);
}
public void updateProgress() {
if (progress < 100) {
steps[progress / 10].setForeground(Color.GREEN);
progress += 10;
progressLabel.setText(progress + "%");
}
}
public static void main(String[] args) {
ProgressMeter meter = new ProgressMeter();
// Set up scanner for user input
Scanner scanner = new Scanner(System.in);
// Listen for user input
while (true) {
System.out.print("Press ENTER to simulate progress: ");
scanner.nextLine();
meter.updateProgress();
}
}
}
```
In this code, the `ProgressMeter` class extends `JFrame` and creates a window with 11 labels - 10 for progress steps and 1 for the progress label. The progress steps are initially red, and the progress label is initially set to "0%".
The `updateProgress()` method is responsible for simulating the progress. It checks if the progress is less than 100, and if so, changes the top-most red label to green, increments the progress by 10, and updates the progress label accordingly. If the progress is already at 100, nothing happens.
The `main()` method sets up a `ProgressMeter` object and listens for user input using a `Scanner` object. Every time the user presses ENTER, the `updateProgress()` method is called to simulate progress.
I hope this helps! Let me know if you have any questions.
相关推荐



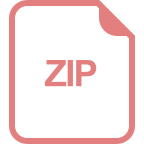
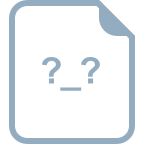
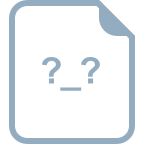
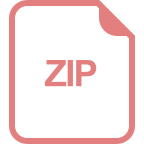