用C语言编写一个猜数游戏,能选择进行人出数计算机猜和计算机出数人猜
时间: 2024-03-02 13:50:18 浏览: 90
下面是一个简单的猜数游戏的 C 语言代码,支持人出数计算机猜和计算机出数人猜两种模式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 计算机出数,人猜
void computer_guess() {
srand(time(NULL));
int number = rand() % 100 + 1;
printf("I have a number between 1 and 100. Can you guess what it is?\n");
int guess, count = 0;
do {
printf("Enter your guess: ");
scanf("%d", &guess);
count++;
if (guess > number) {
printf("Too high! Try again.\n");
} else if (guess < number) {
printf("Too low! Try again.\n");
}
} while (guess != number);
printf("Congratulations! You guessed the number in %d tries.\n", count);
}
// 人出数,计算机猜
void human_guess() {
printf("Think of a number between 1 and 100, and I'll try to guess it.\n");
int low = 1, high = 100, guess, count = 0;
do {
guess = (low + high) / 2;
printf("Is it %d?\n", guess);
printf("Enter '1' if it is too low, '2' if it is too high, or '3' if it is correct: ");
int response;
scanf("%d", &response);
count++;
if (response == 1) {
low = guess + 1;
} else if (response == 2) {
high = guess - 1;
}
} while (guess != number);
printf("I guessed the number in %d tries.\n", count);
}
int main() {
printf("Welcome to the number guessing game!\n");
printf("Enter '1' for computer out and human guess, or '2' for human out and computer guess: ");
int mode;
scanf("%d", &mode);
if (mode == 1) {
computer_guess();
} else if (mode == 2) {
human_guess();
} else {
printf("Invalid mode.\n");
}
return 0;
}
```
在这个程序中,`computer_guess` 函数实现了计算机出数,人猜的游戏模式,而 `human_guess` 函数实现了人出数,计算机猜的游戏模式。在 `main` 函数中,我们首先让用户选择游戏模式,然后根据选择调用相应的函数。在计算机出数,人猜的游戏模式中,我们使用 `rand` 函数生成一个随机数作为计算机所出的数,然后使用一个循环让用户猜测这个数。在人出数,计算机猜的游戏模式中,我们使用二分查找算法让计算机猜测用户所出的数,并根据用户的反馈调整猜测的范围,直到猜中为止。
阅读全文
相关推荐
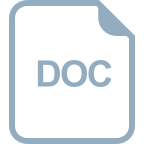
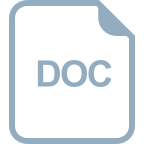
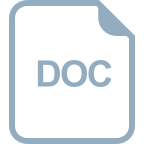
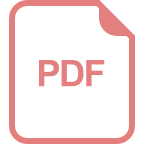


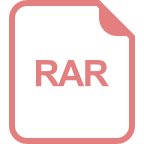
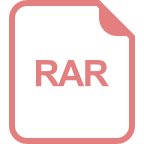
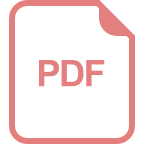
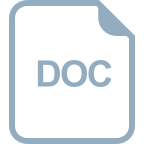
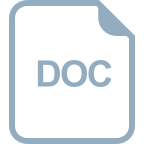
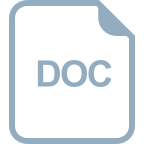
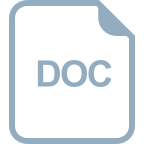





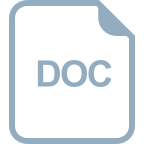