利用Python连接MySQL制作一个学生管理系统代码
时间: 2024-02-24 18:55:34 浏览: 196
好的,下面是一个简单的学生管理系统代码,使用Python连接MySQL数据库实现:
```python
import mysql.connector
# 连接数据库
mydb = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="students"
)
# 创建游标对象
mycursor = mydb.cursor()
# 创建学生表
mycursor.execute("CREATE TABLE students (id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(255), age INT, gender VARCHAR(10), major VARCHAR(255))")
# 插入学生信息
sql = "INSERT INTO students (name, age, gender, major) VALUES (%s, %s, %s, %s)"
val = ("Tom", 20, "Male", "Computer Science")
mycursor.execute(sql, val)
# 查询学生信息
mycursor.execute("SELECT * FROM students")
myresult = mycursor.fetchall()
for x in myresult:
print(x)
# 更新学生信息
sql = "UPDATE students SET major = 'Data Science' WHERE name = 'Tom'"
mycursor.execute(sql)
# 删除学生信息
sql = "DELETE FROM students WHERE name = 'Tom'"
mycursor.execute(sql)
# 提交更改
mydb.commit()
# 关闭连接
mydb.close()
```
需要注意的是,你需要先在MySQL数据库中创建一个名为“students”的数据库,并给自己的用户赋予足够的权限。同时,你需要将代码中的“yourusername”和“yourpassword”替换为你自己的用户名和密码。
阅读全文
相关推荐
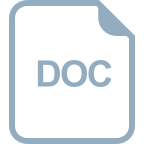
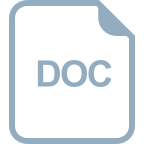
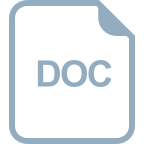
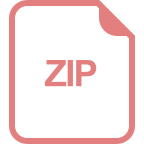
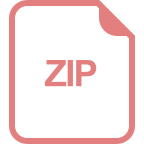
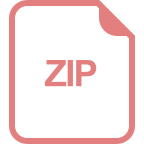
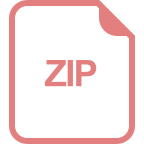
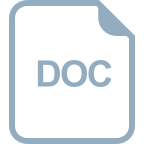
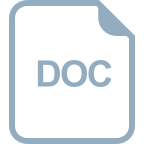
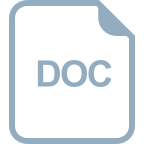
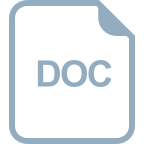
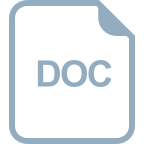
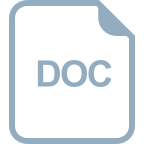
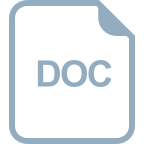
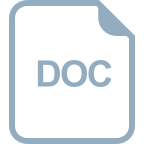
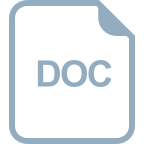
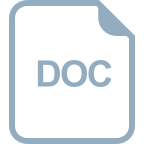
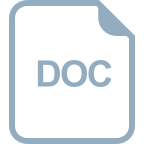
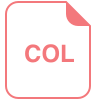
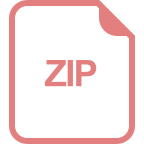