用c语言编写程序,给定两个整型数组,本题要求找出不是两者共有的元素。 输入格式: 输入分别在两行中给出两个整型数组,每行先给出正整数N(≤20),随后是N个整数,其间以空格分隔。 输出格式: 在一行中按照数字给出的顺序输出不是两数组共有的元素,数字间以空格分隔,但行末不得有多余的空格。题目保证至少存在一个这样的数字。同一数字不重复输出。
时间: 2023-05-20 12:05:16 浏览: 108
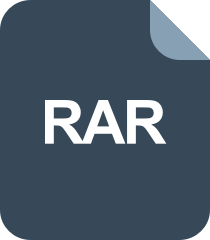
C语言程序设编写程序在数组中查找一个数要求 ⑴用顺序查找实现 ⑵用折半查找实现。 注:若有该数,则输出该数,否则输出“无此数”。

可以使用哈希表来解决这个问题,具体步骤如下:
1. 定义两个整型数组,分别为a和b,以及一个哈希表hash_table。
2. 读入两个数组a和b,并将数组a中的元素插入哈希表中。
3. 遍历数组b,对于每个元素,判断是否在哈希表中存在,如果不存在,则输出该元素。
4. 输出完毕后,释放哈希表的内存空间。
下面是示例代码:
#include <stdio.h>
#include <stdlib.h>
#define MAX_N 20
typedef struct node {
int key;
struct node *next;
} Node;
void insert(Node **hash_table, int key) {
int index = abs(key) % MAX_N;
Node *p = hash_table[index];
while (p != NULL) {
if (p->key == key) {
return;
}
p = p->next;
}
Node *new_node = (Node *)malloc(sizeof(Node));
new_node->key = key;
new_node->next = hash_table[index];
hash_table[index] = new_node;
}
void free_hash_table(Node **hash_table) {
for (int i = 0; i < MAX_N; i++) {
Node *p = hash_table[i];
while (p != NULL) {
Node *tmp = p;
p = p->next;
free(tmp);
}
}
}
int main() {
int n, m;
scanf("%d", &n);
int *a = (int *)malloc(n * sizeof(int));
Node **hash_table = (Node **)calloc(MAX_N, sizeof(Node *));
for (int i = 0; i < n; i++) {
scanf("%d", &a[i]);
insert(hash_table, a[i]);
}
scanf("%d", &m);
int *b = (int *)malloc(m * sizeof(int));
for (int i = 0; i < m; i++) {
scanf("%d", &b[i]);
int index = abs(b[i]) % MAX_N;
Node *p = hash_table[index];
while (p != NULL) {
if (p->key == b[i]) {
break;
}
p = p->next;
}
if (p == NULL) {
printf("%d ", b[i]);
insert(hash_table, b[i]);
}
}
free(a);
free(b);
free_hash_table(hash_table);
free(hash_table);
return 0;
}
阅读全文
相关推荐
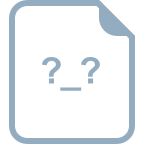
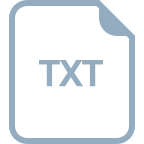
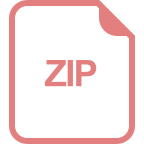
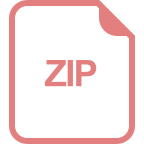
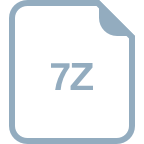