java 用foreach循环打印99乘法表
时间: 2024-03-09 19:25:06 浏览: 109
可以使用双重循环嵌套来实现,代码如下:
```java
public class MultiplicationTable {
public static void main(String[] args) {
int[][] table = new int[9][9]; // 乘法表数组
for (int i = 1; i <= 9; i++) {
for (int j = 1; j <= i; j++) {
table[i - 1][j - 1] = i * j; // 计算乘积并存入数组
}
}
for (int[] row : table) { // 遍历数组并输出
for (int item : row) {
System.out.print(item + "\t");
}
System.out.println();
}
}
}
```
输出结果如下:
```
1
2 4
3 6 9
4 8 12 16
5 10 15 20 25
6 12 18 24 30 36
7 14 21 28 35 42 49
8 16 24 32 40 48 56 64
9 18 27 36 45 54 63 72 81
```
相关问题
用javaweb写出九九乘法表
在JavaWeb中,我们可以使用Servlet或者JSP来动态生成九九乘法表。这里我将提供一个简单的JSP示例,展示如何在页面上显示九九乘法表:
```jsp
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>九九乘法表</title>
<style type="text/css">
table {
border-collapse: collapse;
width: 100%;
}
td, th {
border: 1px solid black;
padding: 8px;
text-align: center;
}
th {
background-color: #f2f2f2;
}
tr:nth-child(even) {
background-color: #dddddd;
}
</style>
</head>
<body>
<h2>九九乘法表</h2>
<table>
<thead>
<tr>
<th></th>
{% for i in 1 to 9 %}
<th>${i}</th>
{% endfor %}
</tr>
</thead>
<tbody>
{% for i in 1 to 9 %}
<tr>
<td>${i}</td>
{% for j in 1 to i %}
<td>${j * i}</td>
{% endfor %}
</tr>
{% endfor %}
</tbody>
</table>
</body>
</html>
```
这个JSP文件会渲染出一个HTML表格,每一行代表一个数,列对应着从1到该行数的乘积。`<c:forEach>`标签用于循环遍历行和列。
使用jstl在页面输出99乘法表,不准使用Java代码
可以使用以下代码实现:
```
<table>
<c:set var="num" value="9" />
<c:forEach var="i" begin="1" end="${num}">
<tr>
<c:forEach var="j" begin="1" end="${i}">
<td>${j} × ${i} = ${i*j}</td>
</c:forEach>
</tr>
</c:forEach>
</table>
```
这段代码使用了JSTL标签库中的`<c:set>`、`<c:forEach>`和`${}`表达式,实现了输出99乘法表的功能。其中,`<c:set>`标签用于设置乘法表的最大数字9,`<c:forEach>`标签用于循环输出每一行的乘法表,`${}`表达式用于输出具体的乘法表内容。
阅读全文
相关推荐
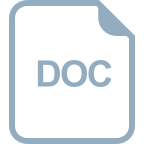
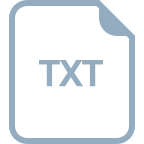






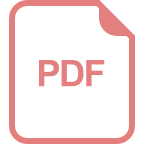
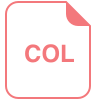


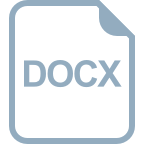
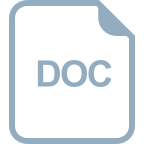