.net怎么使用开源免费库 把word文件转为pdf,请提供代码示例
时间: 2023-08-31 16:44:19 浏览: 64
### 回答1:
您可以使用开源的 iTextSharp 库来将 Word 文件转换为 PDF。
首先,在项目中引用 iTextSharp 库。接着,您可以使用以下代码将 Word 文件转换为 PDF:
```
using iTextSharp.text.pdf;
using iTextSharp.text.pdf.parser;
using System.IO;
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
// 将 Word 文件转换为 PDF
using (FileStream stream = new FileStream("input.docx", FileMode.Open, FileAccess.Read))
{
Document doc = new Document(stream);
PdfWriter.GetInstance(doc, new FileStream("output.pdf", FileMode.Create));
doc.Open();
doc.Add(new Paragraph("Hello World!"));
doc.Close();
}
}
}
}
```
请注意,上面的代码仅适用于将简单的 Word 文件转换为 PDF。如果您要转换复杂的 Word 文件,可能需要使用更复杂的转换方法。
### 回答2:
在.NET中使用开源免费库将Word文件转换为PDF可以通过NPOI库来实现。NPOI是一个在.NET平台上处理Office文档的开源免费库。
首先,我们需要使用NuGet包管理器安装NPOI库。在Visual Studio的解决方案资源管理器中右键单击项目,选择“管理NuGet程序包”。在搜索框中输入“NPOI”,然后点击安装按钮进行安装。
接下来,我们需要引入相关的命名空间和类。
```csharp
using System;
using System.IO;
using NPOI.HWPF;
using NPOI.XWPF.UserModel;
using iTextSharp.text;
using iTextSharp.text.pdf;
```
然后,我们可以编写转换函数。
```csharp
public static void ConvertWordToPdf(string inputPath, string outputPath)
{
using (var fs = new FileStream(outputPath, FileMode.Create, FileAccess.Write))
{
Document doc = new Document();
PdfWriter writer = PdfWriter.GetInstance(doc, fs);
doc.Open();
string extension = Path.GetExtension(inputPath);
if (extension == ".doc")
{
using (var file = new FileStream(inputPath, FileMode.Open, FileAccess.Read))
{
HWPFDocument hwpfDoc = new HWPFDocument(file);
var range = hwpfDoc.Range;
foreach (var paragraph in range.Paragraphs)
{
var elements = paragraph.CharacterRuns;
foreach (var element in elements)
{
var text = element.Text;
doc.Add(new Paragraph(text));
}
}
}
}
else if (extension == ".docx")
{
using (var file = new FileStream(inputPath, FileMode.Open, FileAccess.Read))
{
XWPFDocument xwpfDoc = new XWPFDocument(file);
var paragraphs = xwpfDoc.Paragraphs;
foreach (var paragraph in paragraphs)
{
var elements = paragraph.GetRuns();
foreach (var element in elements)
{
var text = element.Text;
doc.Add(new Paragraph(text));
}
}
}
}
doc.Close();
writer.Close();
}
}
```
最后,我们可以在主程序中调用该函数进行转换。
```csharp
static void Main(string[] args)
{
string inputPath = "input.docx"; // 输入的Word文件路径
string outputPath = "output.pdf"; // 输出的PDF文件路径
ConvertWordToPdf(inputPath, outputPath);
Console.WriteLine("转换完成!");
Console.ReadLine();
}
```
以上代码示例演示了如何使用NPOI和iTextSharp库将Word文件转换为PDF。请注意,为了运行示例,你需要在项目中引用NPOI和iTextSharp库,并替换inputPath和outputPath为实际的文件路径。
### 回答3:
在.NET中,我们可以使用开源和免费的库来将Word文件转换为PDF。一个常用的库是Aspose.Words。以下是一个简单的示例代码:
```csharp
using Aspose.Words;
public static void ConvertWordToPdf(string wordFilePath, string pdfFilePath)
{
// 加载Word文档
Document document = new Document(wordFilePath);
// 保存为PDF格式
document.Save(pdfFilePath, SaveFormat.Pdf);
}
```
在上面的代码中,我们首先通过传递Word文件的路径来加载文档。然后使用Save方法将其保存为PDF格式,并指定输出文件的路径。
要使用Aspose.Words库,您需要先下载并安装该库,并在项目中引用正确的dll文件。您可以在Aspose官方网站上找到该库的下载链接。
请注意,这只是一个简单的示例代码,如果您需要更高级的功能,如设置PDF的页面大小、编码、水印等,您可能需要查看Aspose.Words的文档来了解更多的使用方法。
相关推荐
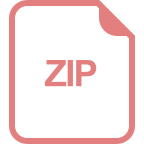
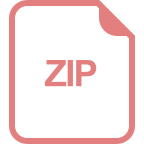
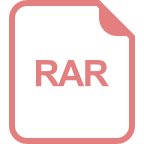












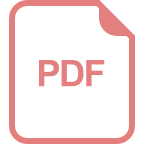
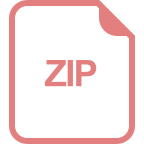