使用JAVA的Swt编写代码,能体现到图形化界面上, 这段代码具体内容能使程序产生一个随机数,然后有用户猜(输入数字),猜中胜,记录花费的时间和猜的次数。猜错提示猜大了还是小了,再继续猜,直到猜对为止。
时间: 2023-11-26 09:02:40 浏览: 55
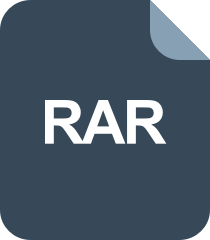
一个用JAVA编写的随机取数小程序

好的,以下是一个基于 SWT 的 Java 程序,实现了你所描述的猜数字游戏:
```java
import org.eclipse.swt.SWT;
import org.eclipse.swt.events.SelectionAdapter;
import org.eclipse.swt.events.SelectionEvent;
import org.eclipse.swt.layout.GridData;
import org.eclipse.swt.layout.GridLayout;
import org.eclipse.swt.widgets.*;
public class GuessNumberGame {
private Shell shell;
private Label titleLabel, promptLabel, resultLabel;
private Text inputText;
private Button submitButton, restartButton;
private int answer, guessCount;
private long startTime;
public GuessNumberGame() {
Display display = new Display();
shell = new Shell(display);
shell.setText("猜数字游戏");
GridLayout layout = new GridLayout(2, false);
shell.setLayout(layout);
titleLabel = new Label(shell, SWT.NONE);
titleLabel.setText("猜数字游戏");
GridData titleData = new GridData(SWT.CENTER, SWT.CENTER, true, false, 2, 1);
titleLabel.setLayoutData(titleData);
promptLabel = new Label(shell, SWT.NONE);
promptLabel.setText("请输入一个 1~100 的数字:");
GridData promptData = new GridData(SWT.CENTER, SWT.CENTER, false, false, 2, 1);
promptLabel.setLayoutData(promptData);
inputText = new Text(shell, SWT.BORDER);
inputText.setLayoutData(new GridData(SWT.FILL, SWT.CENTER, true, false, 1, 1));
submitButton = new Button(shell, SWT.PUSH);
submitButton.setText("提交");
GridData submitData = new GridData(SWT.FILL, SWT.CENTER, false, false, 1, 1);
submitButton.setLayoutData(submitData);
resultLabel = new Label(shell, SWT.WRAP);
resultLabel.setLayoutData(new GridData(SWT.CENTER, SWT.CENTER, true, false, 2, 1)));
restartButton = new Button(shell, SWT.PUSH);
restartButton.setText("重新开始");
GridData restartData = new GridData(SWT.FILL, SWT.CENTER, false, false, 2, 1);
restartButton.setLayoutData(restartData);
restartButton.setVisible(false);
submitButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent selectionEvent) {
try {
int guessNumber = Integer.parseInt(inputText.getText().trim());
if (guessNumber < 1 || guessNumber > 100) {
resultLabel.setText("请输入一个 1~100 的数字!");
} else {
guessCount++;
if (guessNumber == answer) {
long endTime = System.currentTimeMillis();
long duration = endTime - startTime;
resultLabel.setText("恭喜你猜对了!\n共猜了 " + guessCount + " 次,用时 " + duration + " 毫秒。");
inputText.setEnabled(false);
submitButton.setEnabled(false);
restartButton.setVisible(true);
} else if (guessNumber < answer) {
resultLabel.setText("猜小了!");
} else {
resultLabel.setText("猜大了!");
}
}
} catch (NumberFormatException e) {
resultLabel.setText("请输入一个 1~100 的数字!");
}
}
});
restartButton.addSelectionListener(new SelectionAdapter() {
@Override
public void widgetSelected(SelectionEvent selectionEvent) {
startNewGame();
}
});
startNewGame();
shell.pack();
shell.open();
while (!shell.isDisposed()) {
if (!display.readAndDispatch()) {
display.sleep();
}
}
display.dispose();
}
private void startNewGame() {
answer = (int) (Math.random() * 100) + 1;
guessCount = 0;
startTime = System.currentTimeMillis();
inputText.setText("");
resultLabel.setText("");
inputText.setEnabled(true);
submitButton.setEnabled(true);
restartButton.setVisible(false);
}
public static void main(String[] args) {
new GuessNumberGame();
}
}
```
程序会在界面上显示一个输入框和一个按钮,让用户可以输入数字并提交猜测。程序会根据用户猜测的数字与随机生成的答案进行比较,如果猜对了,程序会显示猜测次数和用时,并且禁用输入框和提交按钮,同时显示一个重新开始的按钮。如果猜错了,程序会提示是猜大了还是猜小了,然后让用户继续猜测,直到猜对为止。
阅读全文
相关推荐
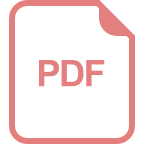
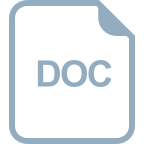
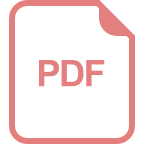
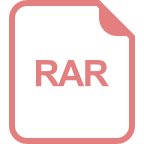
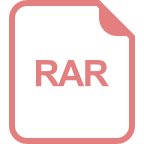
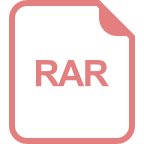
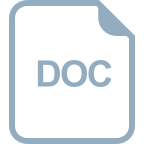
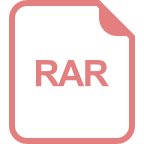
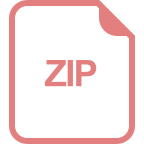