编写一个函数对RGB彩色图片的每一个颜色通道进行二值化
时间: 2023-05-19 16:05:38 浏览: 52
可以使用以下代码对RGB彩色图片的每一个颜色通道进行二值化:
```python
import numpy as np
from PIL import Image
def binarize_image(image_path, threshold):
# Load image and convert to numpy array
image = Image.open(image_path)
image_array = np.array(image)
# Split image into RGB channels
red_channel = image_array[:, :, 0]
green_channel = image_array[:, :, 1]
blue_channel = image_array[:, :, 2]
# Binarize each channel using the given threshold
red_channel[red_channel < threshold] = 0
red_channel[red_channel >= threshold] = 255
green_channel[green_channel < threshold] = 0
green_channel[green_channel >= threshold] = 255
blue_channel[blue_channel < threshold] = 0
blue_channel[blue_channel >= threshold] = 255
# Merge the binarized channels back into a single image
binarized_image_array = np.stack([red_channel, green_channel, blue_channel], axis=2)
binarized_image = Image.fromarray(binarized_image_array)
return binarized_image
```
你可以调用这个函数并传入图片路径和阈值来对图片进行二值化。
相关推荐
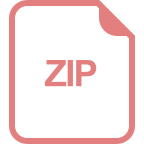
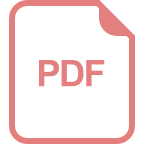














